RxJs In Practice (with FREE E-Book)
Learn numerous RxJs Operators, learn all RxJs and Reactive Programming core concepts via Practical Examples
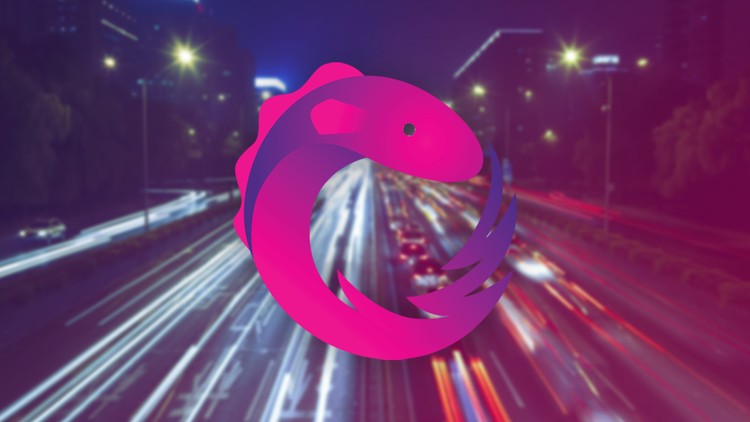
What you will learn
Code in Github repository with downloadable ZIP files per section
Learn the RxJs library via Practical Examples
Become familiar with an extended subset of RxJs Operators
Understand in detail the core notions of Reactive Programming
Learn how to design and build Applications in Reactive style
Why take this course?
🌟 RxJs In Practice (with FREE E-Book) 📚
Master the art of asynchronous data handling with Angular's powerful RxJs library! This comprehensive online course is designed to take you from the basics to mastering numerous RxJs operators through practical examples. 🚀
Course Overview:
-
Introduction to RxJs: Understand the core concepts such as Streams and Observables, and clarify what RxJs is, when to use it, and why it's essential for handling asynchronous operations effectively.
-
Creating Observables from Scratch: Learn how to implement your own Observable from first principles, including handling backend HTTP requests, error handling, and cancellation mechanisms.
-
Practical Operators Explained with Marble Diagrams: Dive deep into the practical usage of operators like
Map
,Filter
,ShareReplay
,concat
,concatMap
,merge
,mergeMap
,exhaustMap
,switch
, andswitchMap
. These examples will cover real-world scenarios such as backend save operations and search typeaheads. -
Error Handling Strategies: Explore various error handling techniques in RxJs, including
catch
,recover
,retry
, and understand how to gracefully handle errors in your applications. -
Reactive Patterns Implementation: Discover how to implement a centralized observable store from first principles using subjects like
BehaviorSubject
andAsyncSubject
. -
A Comprehensive List of Operators: Learn about a wide range of RxJs operators, including but not limited to
withLatestFrom
,forkJoin
,take
,first
,delay
,delayWhen
,startWith
, etc. -
Implement Your Own Custom Operator: Cap off your learning by creating a custom pipeable operator for efficient debugging of RxJS programs.
What You Will Learn:
-
Core Reactive Concepts: Gain a deep understanding of the fundamental concepts that underpin reactive programming with RxJs, such as the differences between imperative and reactive paradigms.
-
Extended Subset of Operators: Master an extended subset of RxJs operators that will cover all your practical needs when building reactive applications with Angular.
-
Practical Application: Learn through real-world examples, ensuring that you can apply your knowledge to create robust and scalable applications using RxJs.
Course Features:
✅ Comprehensive Guide: This course provides a complete, practical guide to the RxJs library for Angular developers.
✅ Extensive Operator Coverage: We cover an extensive subset of operators that will address all your daily needs and help you understand their behavior with official marble diagrams.
✅ Hands-On Learning: Apply what you learn through practical examples, including backend operations, search typeaheads, and more.
✅ Real-World Scenarios: Learn how to implement reactive patterns and debug custom operators in real-world applications.
✅ FREE E-Book Included: The course includes a free e-book to complement your learning experience.
Join us on this journey to become an expert in RxJs and reactive programming with Angular! 🛠️👩💻🚀
Screenshots
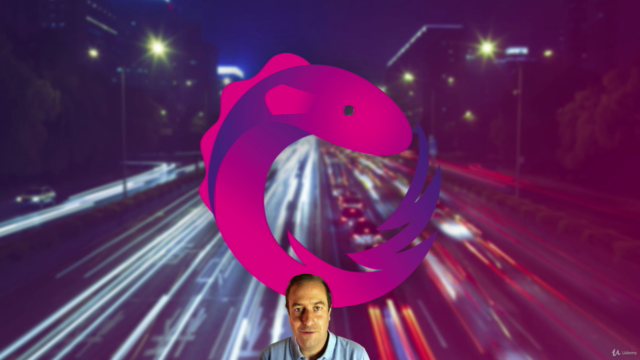
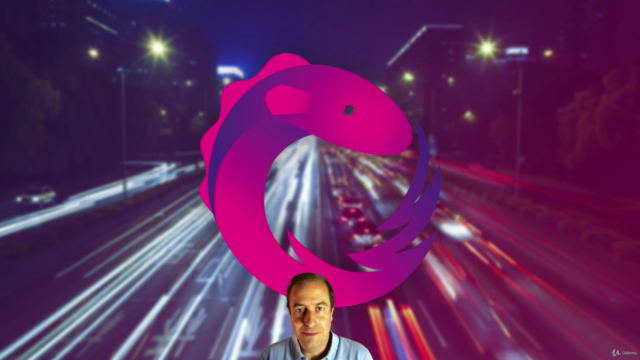
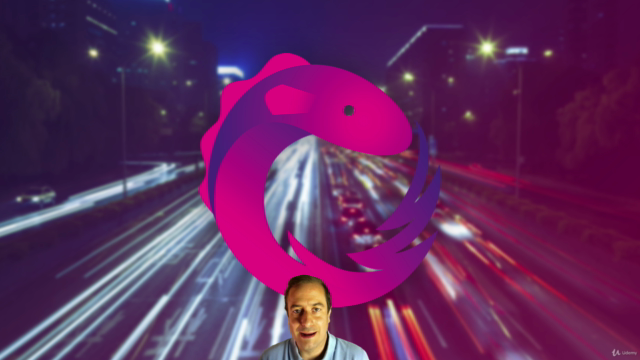

Our review
🧭 Course Overview:
Global course rating: 4.63
Pros:
- 🚀 High Praise for Angular University: Many users have commended Angular University's courses as being the best and most professional on Udemy, especially for Angular-related content.
- 📚 Clear Introductions: The course has been recognized for its clear introduction to the RxJS world, making it a great starting point for beginners unfamiliar with reactive programming.
- 🤝 Knowledgeable Instructor: Vasco has been acknowledged for having an in-depth knowledge of the content and providing clear explanations and practical examples.
- 🎉 Real-Life Application: The course is commended for teaching real-time examples of when to use operators, which helps in understanding the practical use cases in real projects.
- 👍 Foundation Knowledge: Despite some issues with outdated content, the course still offers foundational knowledge that is worth the time and effort for those looking to understand RxJS concepts.
- 🌟 Well-Rounded Introduction: The course provides a comprehensive introduction to RxJs, covering the rationale, basic concepts, operators, and behaviors, according to some user reviews.
- ✅ Good Pacing with Demonstrations: The pacing of the course is generally considered good, with live demonstrations throughout to aid understanding.
Cons:
- 🕰️ Outdated Content: Some users have pointed out that the video content is very old, possibly Angular 6, which can be challenging for beginners, especially when it comes to understanding the newer versions of TypeScript.
- ⬅️ Inconsistent Quality: The course structure has been described as messy and requires a significant amount of tweaking to get the examples to work properly.
- 🤫 Unanswered Questions: There have been instances where student questions remain unanswered after a few years, leading some learners to give up.
- ❌ Deprecated Functions: Some RxJS operators and functions that are officially deprecated are still taught in the course.
- 🛠️ Need for Updates: The course would benefit greatly from updates to reflect the current best practices and remove outdated methods.
- 🤔 Confusing Delivery: Some users found the course delivery to be disorganized, with key concepts being either overly emphasized or rushed through, making it hard to follow along without frequent pauses.
- 📜 Potential for Improvement: User feedback suggests that the inclusion of quizzes and exercises would enhance learning by allowing viewers to test their understanding of the concepts taught in the course.
Additional Notes:
- ❓ Misleading Marketing: Some users have criticized the course for being misleading, as it is advertised as updated but contains outdated content from Angular 6.
- 🎓 Experience Level Discrepancy: The course is marketed as suitable for all experience levels; however, some learners found it to be more of a beginner to intermediate level, which was misleading for those expecting more advanced material.
Final Thoughts:
The course offers valuable content for beginners looking to understand RxJS and reactive programming concepts within the context of Angular. However, potential students should be aware that some parts of the course are outdated and may not align with current best practices. Users have requested updates and a more structured approach with interactive elements like quizzes and exercises to enhance the learning experience. The positive feedback on the depth and clarity of instruction from Vasco indicates that with updated content, this could be a standout course in the Angular community.