Python 3: Fundamentals
Learn Python the right way!
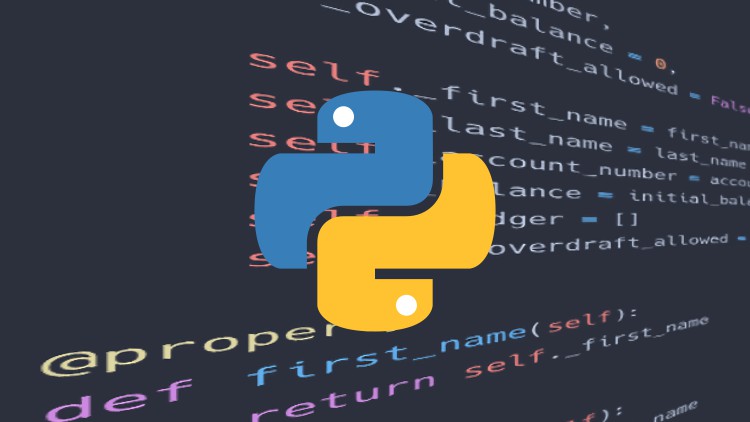
What you will learn
Learn Python fundamentals
Basic to advanced data types
Functional and Object Oriented programming
Closures and decorators
Datetime and timezone handling
Reading and writing text, CSV and JSON files
Making REST API requests
Fundamentals of NumPy
Fundamentals of Pandas
Fundamentals of Matplotlib
Why take this course?
This Course in a Nutshell
Aimed at beginner Python developers, this course will provide you a fundamental understanding of how to program in Python. Your journey will take you from a total Python beginner to an intermediate level Python developer more than ready to tackle your own professional projects.
Although not technically required, limited prior experience in any coding language, and a basic understanding of concepts such as looping, branching, etc will prove useful.
This course will provide you the solid foundation you will need to continue moving forward in your Python development endeavors. This course is not a "here's how I do it, just type along please" type of course - its goal is to make you understand each and every line of code we are going to write together, why we write it and why it works, giving you the knowledge to apply the same coding techniques to your own situation and problems.
Like any good college level course, this course is fairly lengthy and will require time, not only for watching the videos, but also working on your own to explore the various topics, trying things out, and at the end of each section working on increasingly complex problems. It takes a certain amount of time to master a programming language, and this course is no exception. If you are looking for a quick and superficial intro to Python, then this course is probably not for you.
VERY IMPORTANT: Before attempting this course you must have a basic knowledge of how to use the DOS prompt (Windows) or shell (Linux/Mac). This means how to open a prompt/shell on your computer, navigating the file structure using cd, creating and deleting directories, copying files/directories from one location to another, listing files in the current directory, etc. There are plenty of 20-30 minute tutorials available online that will teach you those simple basics.
Course Overview
This course balances theory and coding practice. Most subjects are two-part: a theory (or lecture) video where we cover a specific topic, explain how things work, followed by a practice (or coding) video which takes the lecture material and applies it using real code. I highly encourage you to take notes during the lectures, and code along with me during the coding videos - that's the beauty of online videos - you can pause, rewind, speed up, slow down as you need!
All the course slides (over 900 of them!) are available for download if you prefer that approach over taking your own notes - however I recommend you take your own notes, preferably after watching the corresponding code video - you'll remember things better that way!
We use Jupyter notebooks as the perfect tool for teaching and learning Python.
Jupyter notebooks support both Python code as well as interspersed markdown documentation. You will find that every code video in this course has a corresponding Jupyter notebook available in the course downloads, that not only reproduces all the code we do in the coding videos, but is fully annotated with explanations of the code, basically what I cover in the coding videos, and sometimes more!
All the notebooks and the data files we will work with, are available in the course downloads in the first section of this course as well as in GitHub.
At the end of each section, you will find a set of exercises with solutions. It is imperative that you work through these exercises, and only move on to the next section once you are able to do these exercises on your own. Each section of this course builds on top of the previous one!
The course is broadly broken down into three main parts:
Python Basics
What is Python
How to install Python
How to create and use virtual environments
How to run Python and Jupyter notebooks
Basic data types including integers, floats, booleans
Boolean operators
Arithmetic and comparison operators, as well as operator precedence
Conditional execution
Looping (for and while)
Sequence types such as lists, tuples and strings
Working with sequence types (iterating, slicing, manipulating, copying, unpacking)
More on strings and Unicode
Dictionaries and sets
Python's list, dictionary and set comprehensions
Exceptions and exception handling
Iterables and iterators, including generators
Writing user defined functions and different ways of defining and passing arguments
Lambda functions
Some of Python's built-in functions (such as zip, sorted, min, max, and round)
Intermediate Python
Higher order functions (passing and returning functions from functions)
Maps (dictionaries)
Closures
Advanced sorting and filtering
Decorators - what they are, and how to write your own
Reading and writing text files
Python's module and import system
How to work with dates and times
How to read and write CSV files
Random numbers and sampling
A look at Python Math and Stats modules
Decimal data type - for when floats aren't precise enough
How to write your own custom Classes (OOP)
3rd Party Libraries
the pytz library for dealing with timezones and daylight savings
the dateutil library for parsing date/time strings
What is JSON data, and how to read and write JSON
What are REST APIs
How to use the requests library for HTTP/s requests (and how to interact with a REST API)
Fundamentals of the NumPy library for fast numerical computations
Fundamentals of the Pandas library for working with data sets (including indexing)
Fundamentals of the matplotlib library for charting data
Reviews
Charts
Price
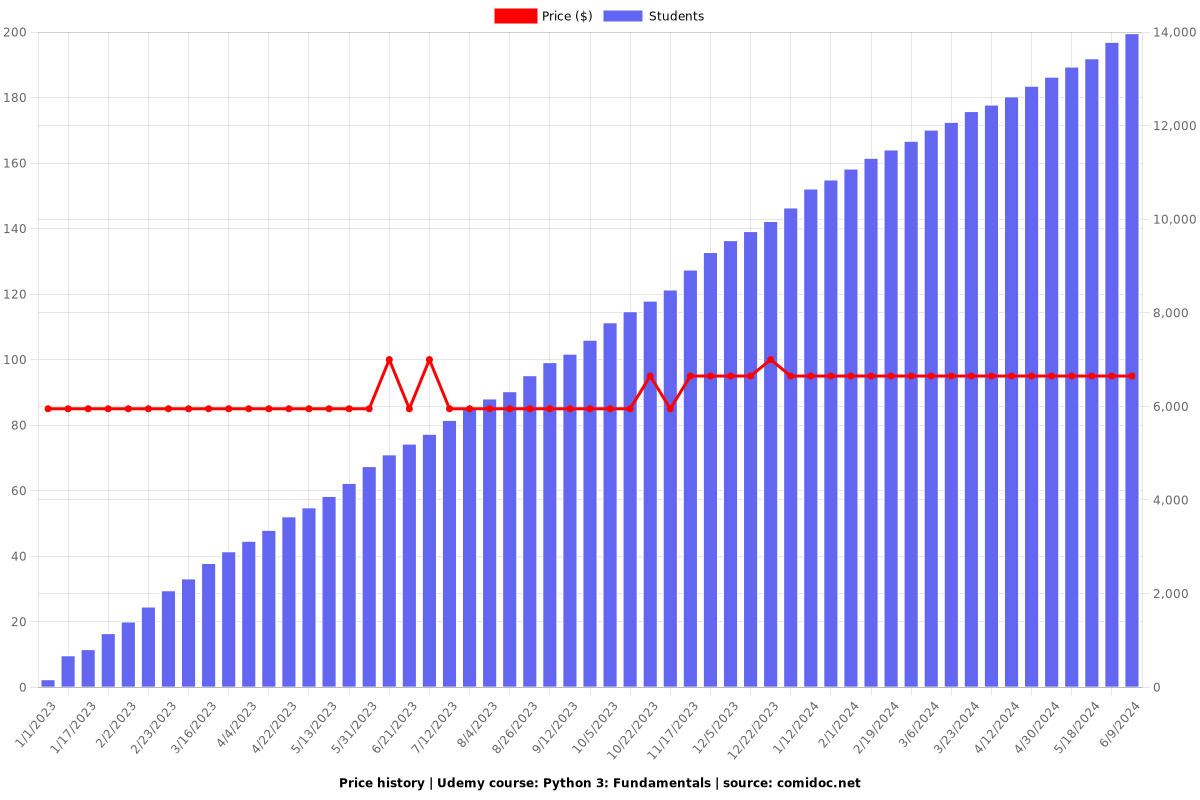
Rating
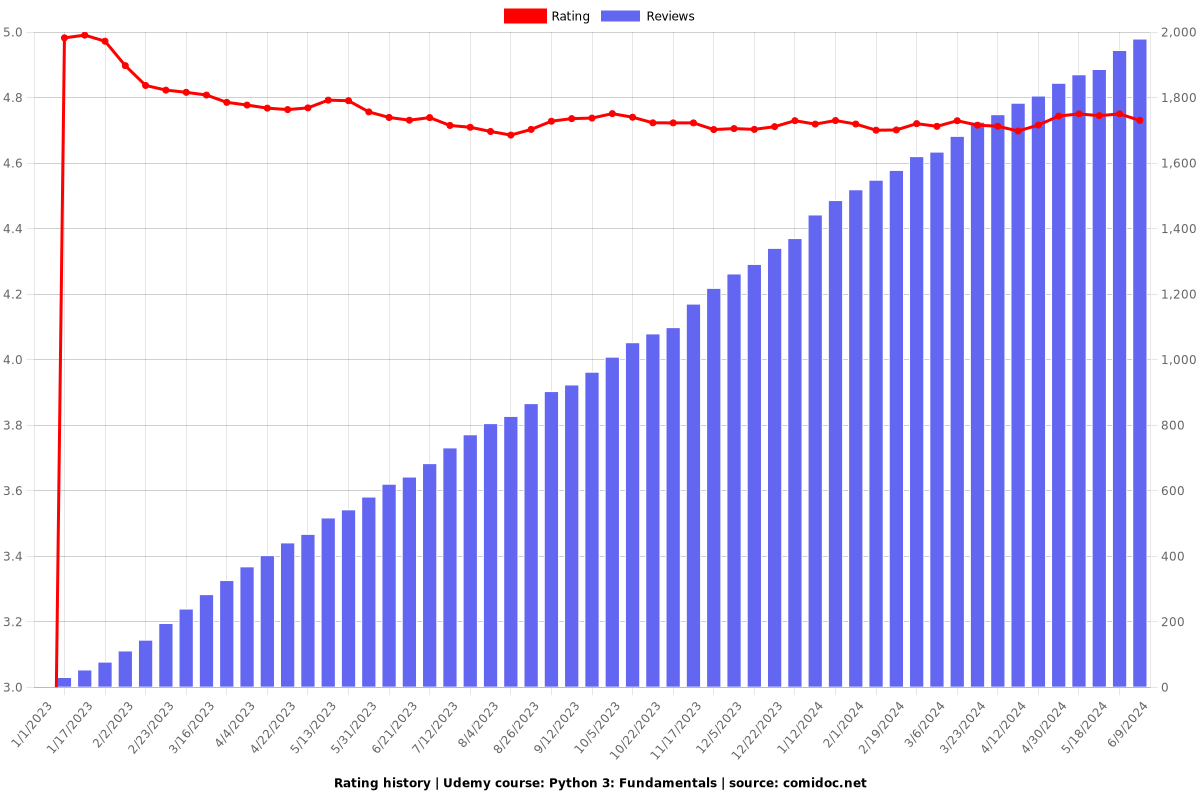
Enrollment distribution
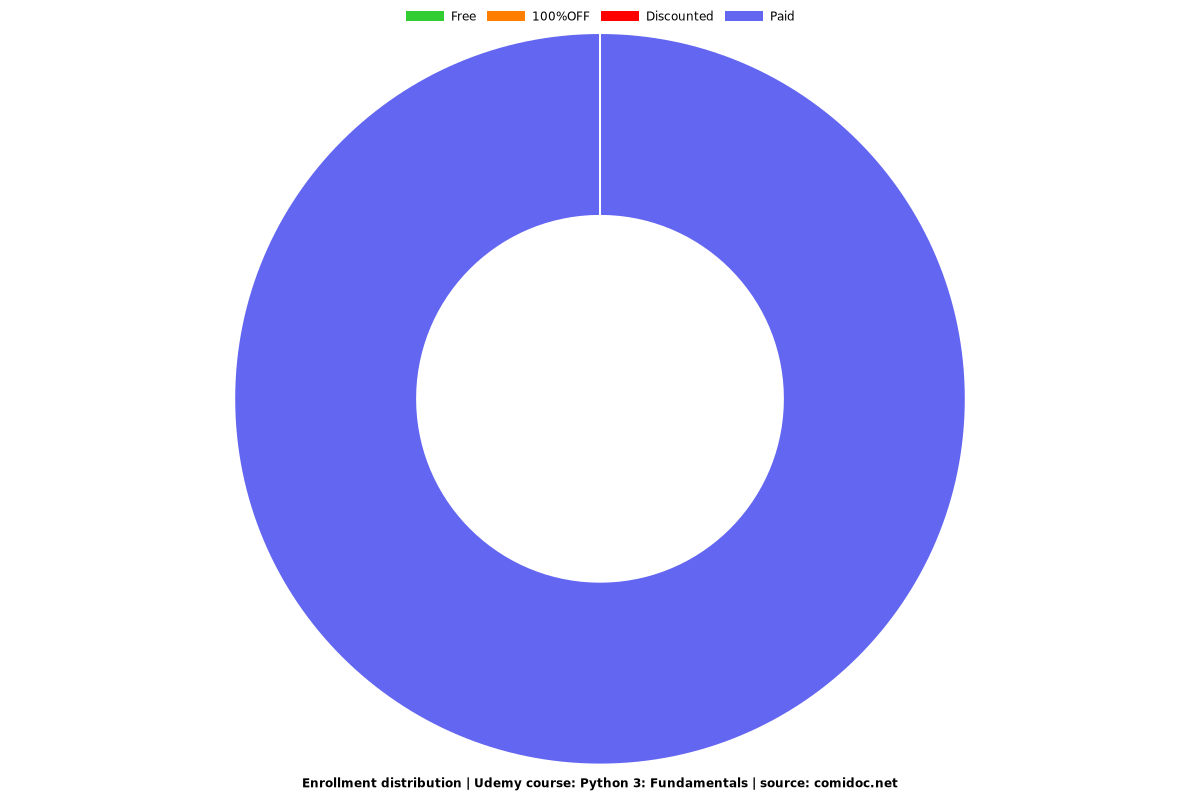