Title
Comprehensive C++ Programming Practice Test: Code Mastery
Comprehensive C++ Programming Practice Test Challenge: Test Your Knowledge with Practice Questions
4.50 (2 reviews)
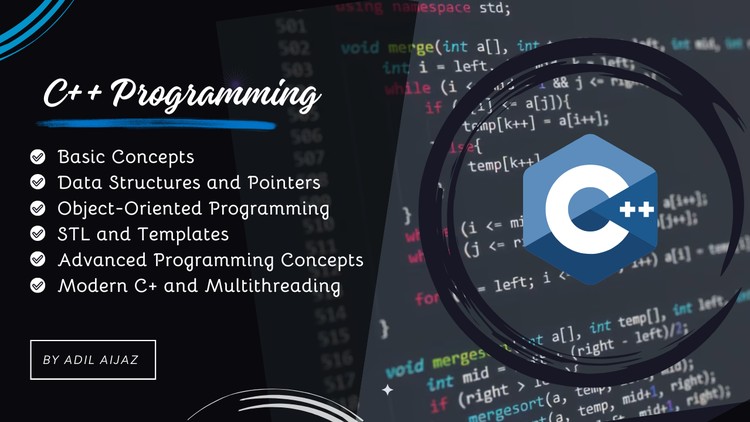
2 453
students
140 questions
content
Oct 2024
last update
$44.99
regular price
What you will learn
Strengthen foundational understanding of C++ basics, including syntax, data types, and operators.
Develop a solid grasp of object-oriented programming concepts like classes, inheritance, and polymorphism.
Practice advanced programming techniques with templates, exception handling, and file handling.
Understand and apply memory management techniques using pointers, smart pointers, and dynamic memory allocation.
Explore and implement modern C++ features, including lambdas, move semantics, and the Standard Template Library (STL).
Gain practical experience with multithreading, concurrency, and C++’s support for parallel processing.
Apply C++ concepts through a variety of question types, including coding-focused MCQs, multiple selections, and true/false.
Why take this course?
📚 Section 1: Basic Concepts of C++
- Data Types, Variables, and Declarations: Learn about the fundamental data types (int, float, char, etc.), variable declarations, and their memory representations.
- Control Structures: Understand how to use conditional statements (if, else), loops (for, while), and jump statements (break, continue, goto) to control the flow of your program.
- Functions: Explore function definitions, return types, arguments (by value or by reference), function overloading, default parameters, and the ability to return references to local variables (return *w;).
🎭 Section 2: Object-Oriented Programming in C++
- Classes and Objects: Dive into object-oriented programming (OOP) concepts by defining classes, creating objects as instances of classes, and using constructors for initialization.
- Encapsulation: Learn how to use access specifiers (public, protected, private) to encapsulate data and member functions within a class.
- Inheritance: Understand how to derive new classes from existing ones, use inheritance to form hierarchies of related classes, and explore the concept of polymorphism.
- Polymorphism: Explore polymorphism through function overriding and virtual functions, which allows different classes to respond to the same message in different ways.
- Overloading and Overriding: Learn how to overload functions (i.e., having multiple functions with the same name but different parameters) and override functions (i.e., redefining a function already defined in a base class).
- Abstract Classes and Interfaces: Understand abstract classes that cannot be instantiated and must be derived from, and how they define an interface for their subclasses.
- Composition vs. Inheritance: Decide when to use composition or inheritance based on the relationship between classes.
🧠 Section 3: Core Language Features
- Template Metaprogramming: Learn how to perform operations at compile time rather than runtime using templates, which can greatly optimize performance for algorithms and data structures.
- Type Casting and Conversion: C++ allows both implicit and explicit type casting. You’ll learn when type conversion is automatic and how to use static_cast, dynamic_cast, and other casting operators for precision in data handling.
🌍 Section 4: Memory Management in C++
- Pointers and References: Pointers point to memory addresses, and references provide aliases for variables. You’ll practice using pointers for memory management, understanding dereferencing, and avoiding null pointer issues.
- Dynamic Memory Allocation: Using
new
anddelete
, C++ allows manual memory allocation. Questions cover dynamic arrays, memory allocation for large data structures, and how to prevent memory leaks. - Smart Pointers: Smart pointers, like
unique_ptr
,shared_ptr
, andweak_ptr
, manage memory automatically. You’ll understand how each smart pointer type works and why they’re essential in modern C++ for safe resource management. - Memory Leaks and Prevention: Memory leaks can degrade program performance. You’ll explore causes of memory leaks and learn best practices to prevent them, such as using smart pointers and deallocating memory properly.
- Pointer Arithmetic and Safety: With pointers, you can directly access and manipulate memory. Questions focus on pointer arithmetic, understanding how pointer addresses shift, and practicing safe memory access to avoid crashes.
🛠️ Section 5: Advanced Programming Concepts
- Exception Handling and Error Management: This topic builds on earlier exception handling, focusing on more complex scenarios. You’ll explore handling multiple exceptions, chaining exceptions, and creating custom exception classes.
- File Handling: Reading from and writing to files is crucial for many applications. Questions cover using
ifstream
andofstream
to open files, handle file streams, and process data stored externally. - Operator Overloading: Operator overloading lets you customize operators for classes. You’ll learn to overload arithmetic operators for complex data structures, giving custom classes more intuitive behavior.
- Type Casting and Conversion: Building on earlier material, you’ll examine different casting operators like
const_cast
,reinterpret_cast
, anddynamic_cast
, practicing precise control over data type conversions.
🌫️ Section 6: Modern C++ and Multithreading
- Smart Pointers: A deeper dive into smart pointers for better resource management, including understanding
shared_ptr
's thread-safe reference counting andstd::unique_ptr
's single ownership semantics. - Concurrency and Parallelism: Understand the pthread library for creating native threads, how to use the C++11
<thread>
library, and synchronization mechanisms like mutexes, condition variables, atomic variables, and barriers. - Lambda Expressions and Closures: Learn how to capture local variables and create inline anonymous functions that can be used as parameters or in algorithms.
- STL (Standard Template Library): Get hands-on experience with the powerful STL containers like
vector
,map
,set
, etc., and their iterators, as well as algorithms for tasks like searching, sorting, filtering, and transforming data. - C++11 and Beyond: Explore modern C++ features such as range-based for loops, nullptr, auto keyword, initialization lists,
constexpr
, lambdas, smart pointers (unique_ptr
,shared_ptr
), and concurrency libraries. - Memory Ordering and Memory Model: Understand the various memory ordering guarantees provided by C++ and how they impact the predictability of code behavior in a multi-threaded context.
- C++20 Features: Get familiar with the new features introduced in C++20, such as concepts, ranges, coroutines, concept-based constexpr lambdas, and more.
🚀 Practice Makes Perfect
- Practice coding exercises to solidify your understanding of C++ concepts.
- Participate in coding challenges and hackathons to apply your skills in real-world scenarios.
- Contribute to open-source projects to gain experience with collaborative development and different coding styles.
- Engage with the community through forums, meetups, and conferences to stay updated on best practices and new developments in the C++ ecosystem.
By mastering these areas, you'll become proficient in C++ and be well-equipped to tackle complex software development challenges! 🚀✨
Charts
Price

Rating

Enrollment distribution

Coupons
Submit by | Date | Coupon Code | Discount | Emitted/Used | Status |
---|---|---|---|---|---|
Angelcrc Seven | 29/10/2024 | CPLUSPLUS | 100% OFF | 1000/985 | expired |
- | 23/11/2024 | CPLUSPLUSFREE | 100% OFF | 1000/283 | expired |
Angelcrc Seven | 16/12/2024 | CPLUSPLUSPROG | 100% OFF | 1000/533 | expired |
- | 17/02/2025 | CPLUSPLUSTEST | 100% OFF | 1000/115 | expired |
6252731
udemy ID
24/10/2024
course created date
29/10/2024
course indexed date
Angelcrc Seven
course submited by