Build Production Ready REST API in Spring Boot - Expense App
Learn building production ready REST APIs in Spring Boot 3, JPA, Spring Security 6, JWT, MySQL and Deploy to AWS, Docker
4.45 (386 reviews)
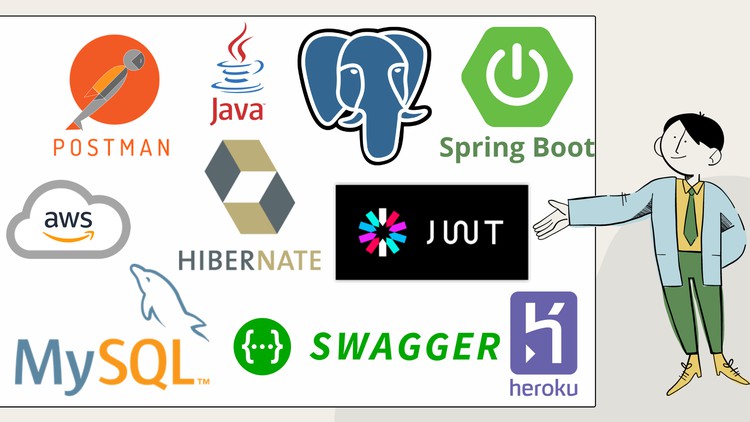
4,685
students
22 hours
content
Mar 2025
last update
$13.99
regular price
What you will learn
Learn building End-to-End Production Ready REST API
Build a complete Expense Manager REST API with Spring Boot, Spring Security, Data JPA, JWT and MySQL Database
Learn to create REST end points to perform Database operations
Learn how validate the REST APIs
Learn how to handle Exceptions and create Custom Exceptions
Learn how create Mapping between two Entities
One to One mapping
One to One Bi-directional mapping
One to Many mapping
One to Many Bi-directional mapping
Learn how to filter the records by writing JPA finder/query methods
Learn how to use Lombok
Learn how use Spring Security in Spring Boot application
Learn how to configure multiple Users using In-memory Authentication
Learn how to test REST APIs in Postman effectively
Learn how to use Postman advance features
Learn how to add Swagger for API Documentation
Learn how to add JWT token based Authentication to Spring Boot application
Learn how to deploy Spring Boot application to Heroku
Learn how to deploy Spring Boot application to AWS
Learn how to dockerize the Spring Boot Application with MySQL database
Learn how to Create REST API with Spring Boot and MongoDB database
Learn how to connect Spring Boot application with MongoDB database
Learn how to Perform the database (CRUD) operations with MongoRepository
Learn how to create finder methods using MongoRepository
Learn how to connect Spring Boot application with MongoDB Atlas (Production Server)
And many more...
Screenshots
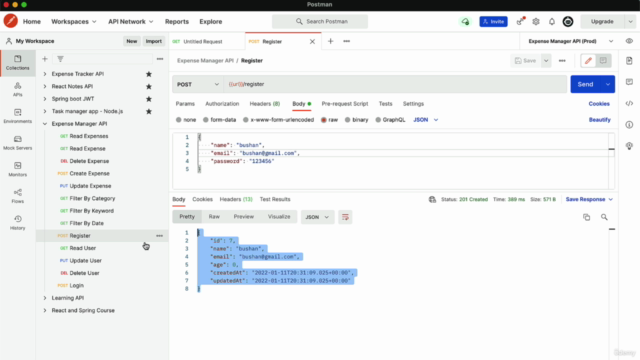
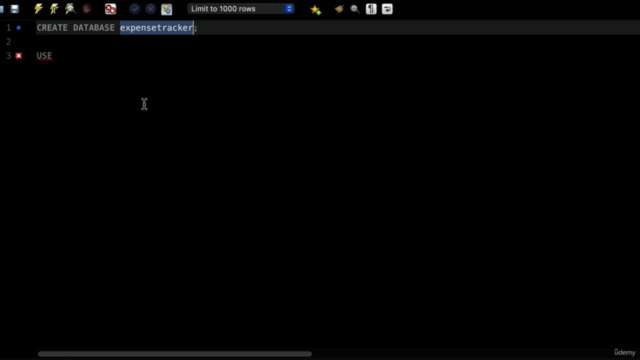
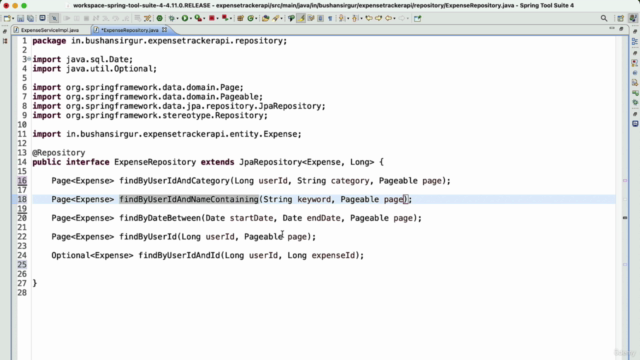
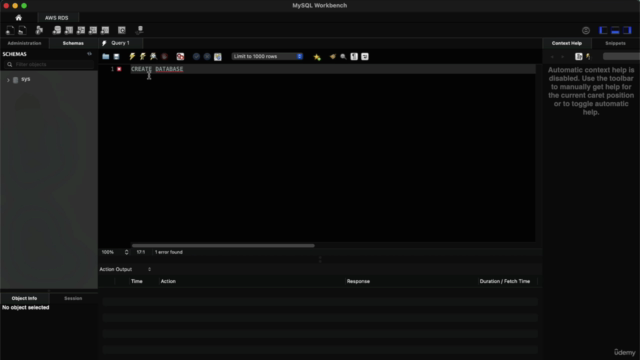
Related Topics
4046252
udemy ID
5/13/2021
course created date
1/14/2022
course indexed date
Bot
course submited by