Algorithms Data Structures in Java #1 (+INTERVIEW QUESTIONS)
Basic Algorithms and Data Structures: AVL tree, Binary Search Trees, Arrays, B Trees, Linked Lists, Stacks and HashMaps
4.58 (2964 reviews)
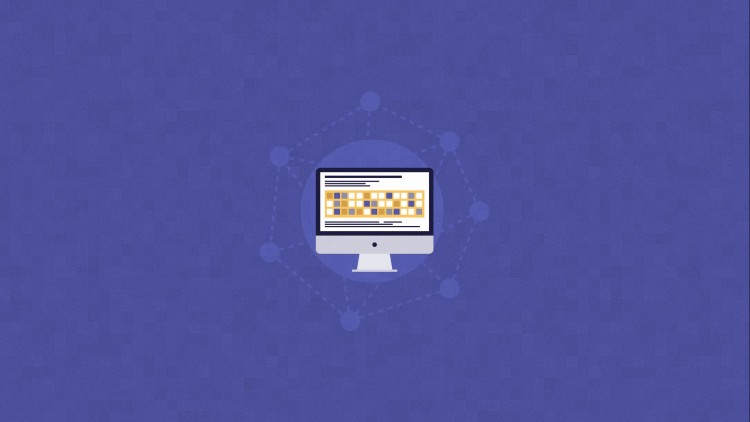
26,741
students
23 hours
content
Mar 2024
last update
$94.99
regular price
What you will learn
grasp the fundamentals of algorithms and data structures
detect non-optimal code snippets
learn about arrays and linked lists
learn about stacks and queues
learn about binary search trees
learn about balanced binary search trees such as AVL trees or red-black trees
learn about priority queues and heaps
learn about B-trees and external memory
learn about hashing and hash tables
Screenshots
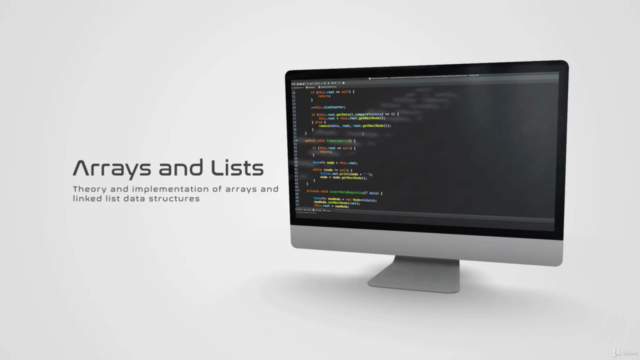
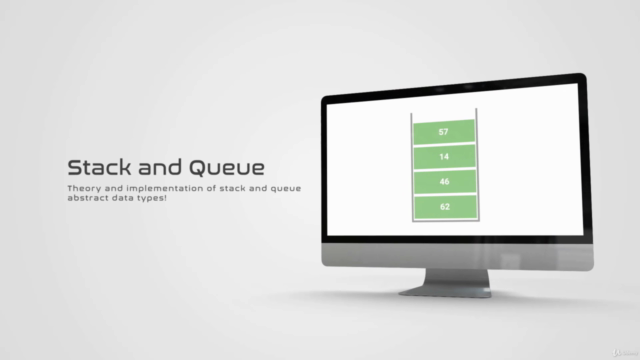
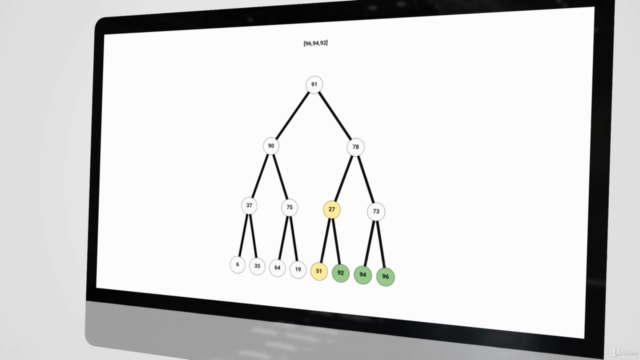
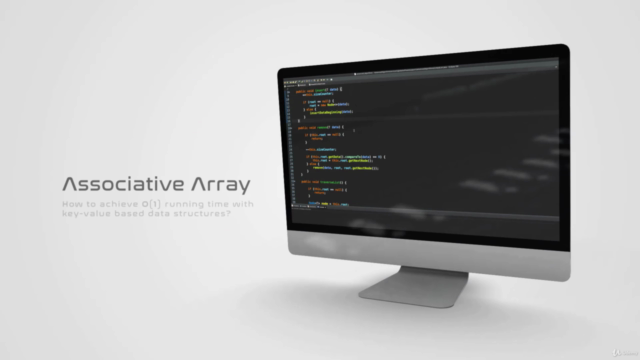
Related Topics
433280
udemy ID
2/26/2015
course created date
11/21/2019
course indexed date
Bot
course submited by