Intermediate to Advanced Python with 10 OOP Projects
Everything you need to know to become an expert in Python including OOP, Git, APIs, databases, deployment, PEP8 and more
4.55 (3745 reviews)
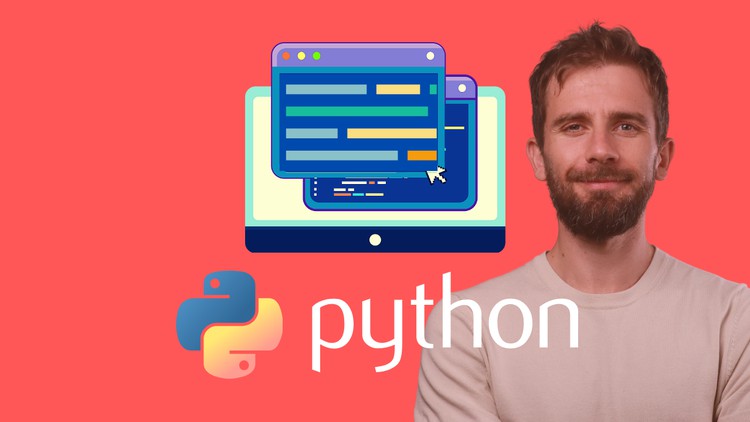
38,044
students
23.5 hours
content
Jan 2025
last update
$129.99
regular price
What you will learn
Master Object-Oriented Programming (OOP) in Python
Build 10 Python applications using object-oriented programming
Learn databases, APIs, package development, Git, code refactoring, debugging, and more
Build three advanced Python projects on your own
Learn to write high quality code following the PEP8 Style Guide
Learn how to deploy applications making them accessible to users globally
Screenshots
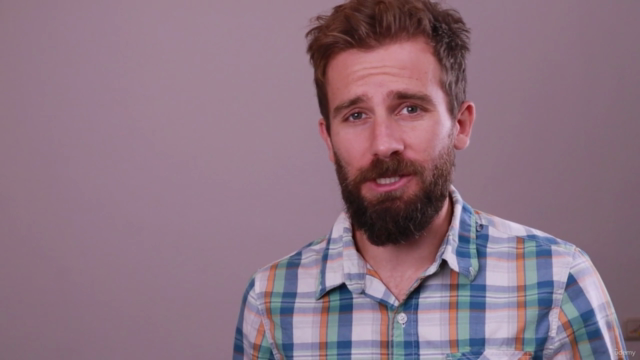
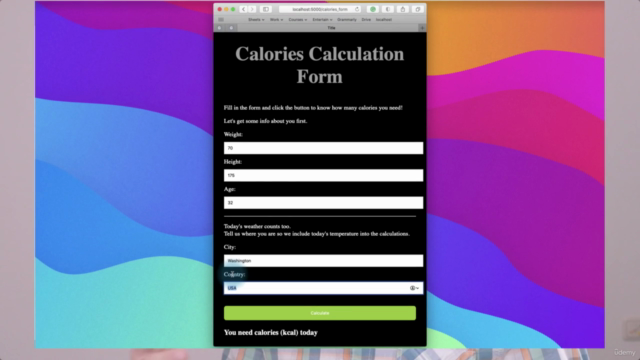
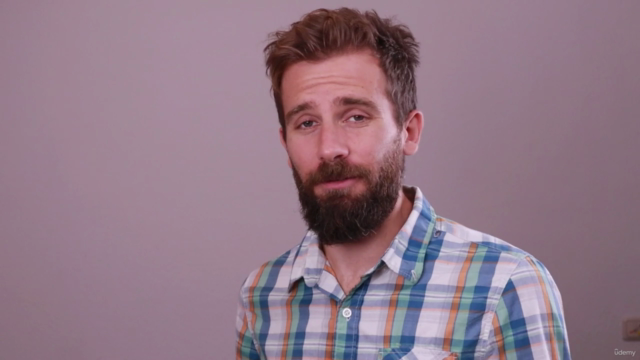
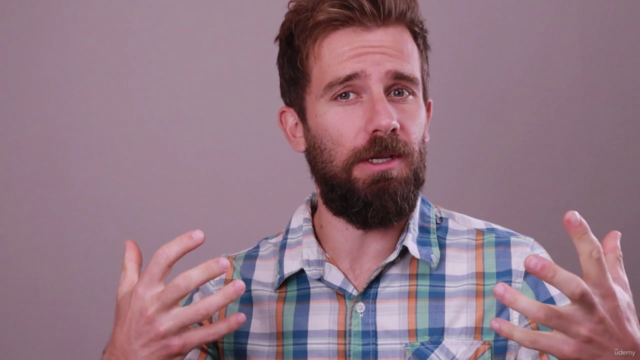
2563788
udemy ID
9/17/2019
course created date
1/14/2021
course indexed date
Bot
course submited by