C# Mastery: Comprehensive Beginner to Advanced Training
Unlock the full potential of C# programming from beginner to advanced levels with our comprehensive training course.
4.18 (260 reviews)
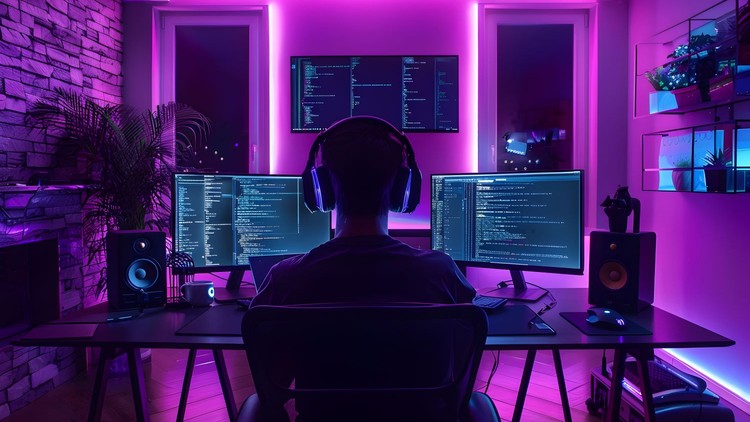
41,539
students
24 hours
content
Mar 2024
last update
$19.99
regular price
What you will learn
Introduction to C# programming language and its structure.
Basics of C# programming including variable declaration, data types, and operators.
Conditional and loop constructs for decision-making and repetition.
Working with arrays and understanding object-oriented programming (OOP) concepts.
Implementing methods, constructors, and static members in C#.
Inheritance, polymorphism, and abstraction in OOP.
Handling exceptions and implementing multithreading for concurrent execution.
File I/O operations, including reading from and writing to files.
Advanced topics such as delegates, events, and lambda expressions.
Introduction to LINQ (Language Integrated Query) and asynchronous programming.
Building applications with WPF (Windows Presentation Foundation) and ASP .NET.
Exploring async and await keywords for asynchronous programming in C#.
Screenshots
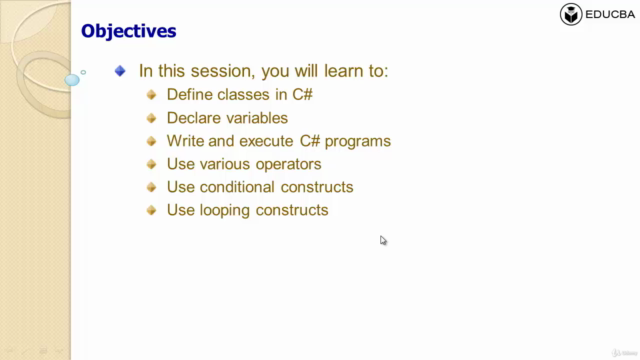
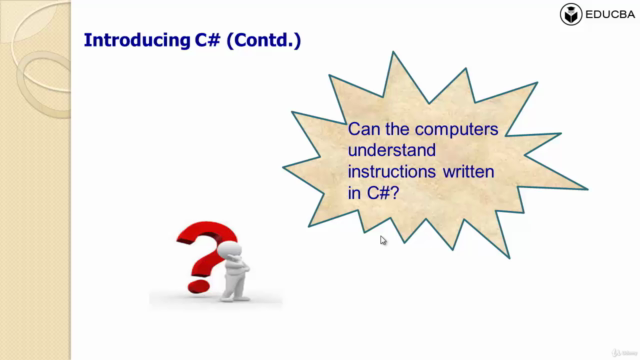
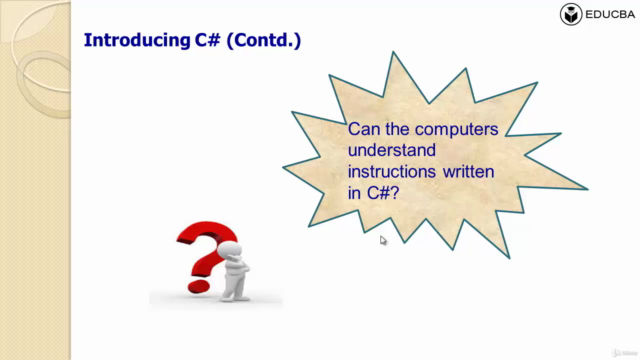
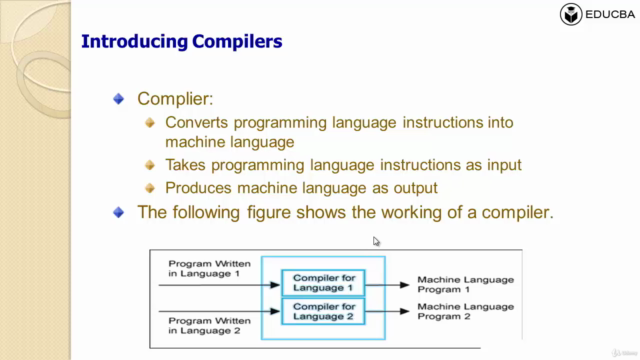
Related Topics
1981652
udemy ID
10/22/2018
course created date
5/29/2019
course indexed date
Bot
course submited by