Spring Boot Testing with JUnit 5, Mockito & Testcontainers
Unit tests and Integration tests for Spring Boot App and Spring WebFlux App using JUnit 5, Mockito and Testcontainers
4.49 (1555 reviews)
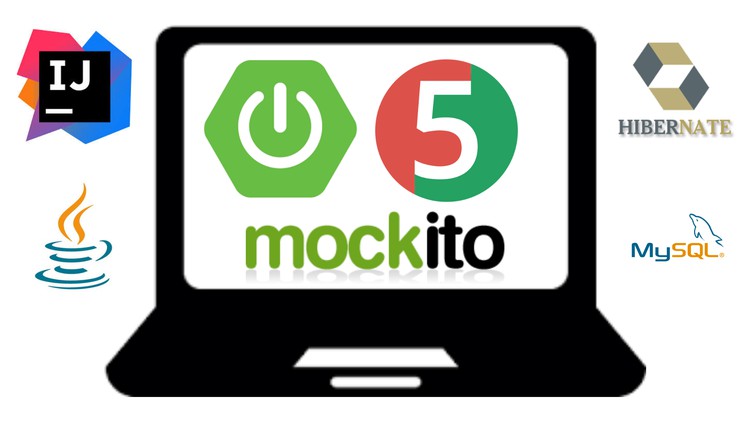
11,956
students
16 hours
content
Mar 2025
last update
$84.99
regular price
What you will learn
Learn to write industry-standard Unit and Integration tests in BDD (Behaviour Driven Development) style using Spring Boot Starter Test dependency from scratch
Learn how to use BDD (Behaviour Driven Development) format that is given/when/then to write Unit tests.
Learn everything about JUnit Framework 5
Learn to Unit test Spring boot application Repository layer
Learn to Unit test Spring boot application Service layer
Learn to Unit test Spring boot application Controller layer - Unit test REST API's
Learn how to do Integration testing for the Spring boot application
You will learn to use the most important Unit Testing annotations - @SpringBootTest, @WebMvcTest, @DataJpaTest, and @MockBean
Use all the frameworks in Spring Boot Starter Test - JUnit, Spring Test, Spring Boot Test, AssertJ, Hamcrest, Mockito, JSONassert, and JsonPath.
You will learn to write Unit tests using Mocks and Stubs created with Mockito
Learn how to use Mockito annotations to create mock objects.
Learn to write Integration Tests using a MySQL database
You will learn to write independent Integration tests for RESTFUL web services talking with MULTIPLE LAYERS - controller, service, and repository layers.
Learn to write Integration Tests using a Testcontainers
Learn Building Reactive CRUD REST APIs using Spring WebFlux and MongoDB
Learn Unit Testing Reactive CRUD REST APIs using JUnit and Mockito
Learn Integration Testing Reactive CRUD REST APIs using WebTestClient
Screenshots
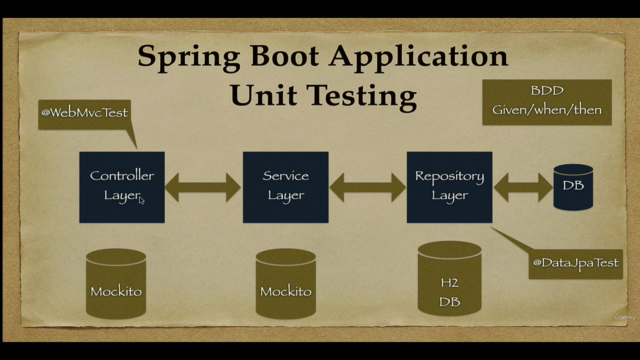
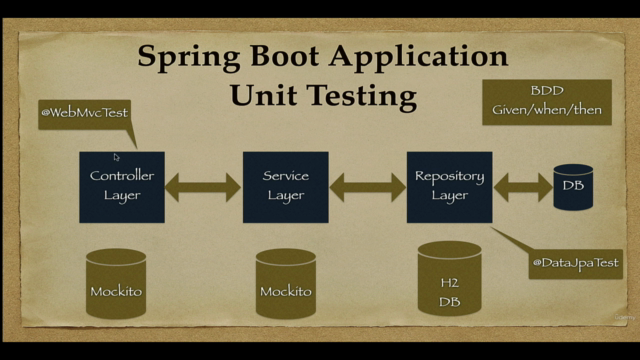
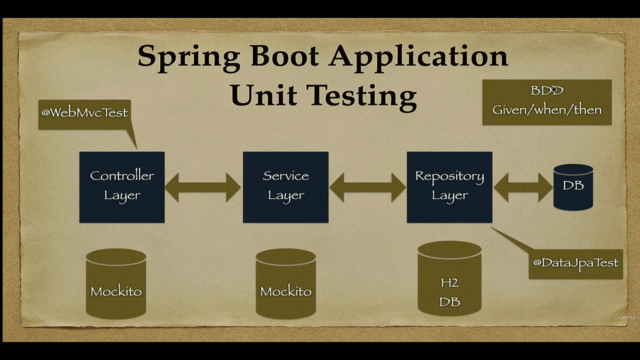
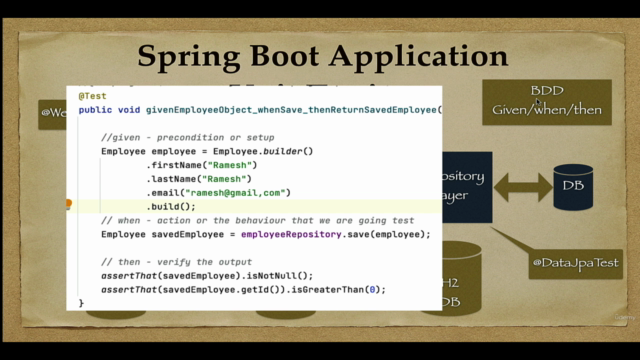
Related Topics
4457400
udemy ID
12/23/2021
course created date
12/30/2021
course indexed date
Bot
course submited by