Test Driven Development in ASP.NET Core - The Handbook
Get Hands-On experience using Unit testing to produce a bullet proof and testable N-Tier .NET Core application.
4.51 (430 reviews)
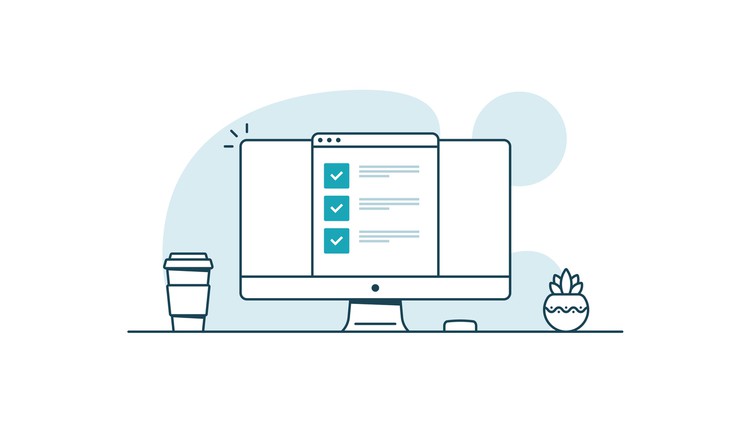
5,437
students
4.5 hours
content
Nov 2024
last update
$69.99
regular price
What you will learn
Unit Testing - Testing Core Application Code
Moq Framework
xUnit Testing Framework
Integration Testing - Testing Third Library Interactions (Like EF Core)
Application Level Unit Testing - Testing that the MVC Application behave correctly
Test Driven Development - Red-Green-Refactor Cycle
Using Facts and Theories to write tests
How to write testable code
Know about good unit testing patterns and practices
Unit testing in N-tier web application projects using xUnit
Continuous Integration and Git Branch Protection
4235116
udemy ID
8/11/2021
course created date
9/16/2021
course indexed date
Bot
course submited by