Become a Front End Web Developer - JavaScript for Beginners
Best JavaScript course for beginners with step by step instructions. Learn basics, ES6, DOM and AJAX with mini project.
4.43 (303 reviews)
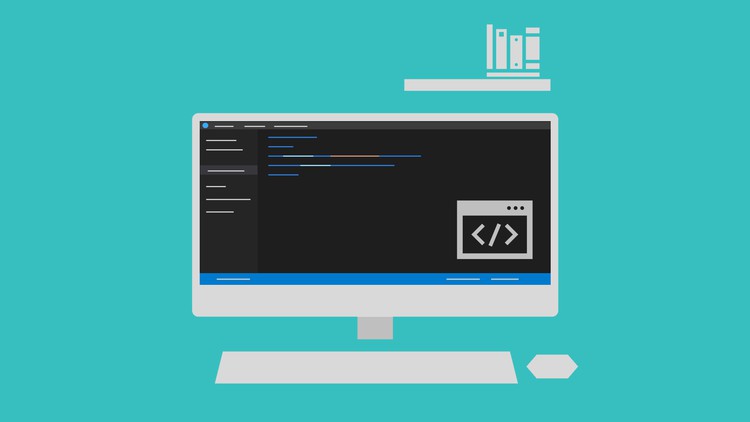
25,878
students
15.5 hours
content
Feb 2022
last update
$44.99
regular price
What you will learn
Fine grained steps and instructions to learn JavaScript
Learn statements and code execution flow
Learn to implement mathematical calculations using Literals and Arithmetic Operators
Learn to use Variables
Learn code reusability using Functions
Learn to implement decision making using Conditional Statements
Learn to repeat statements using Looping constructs
Learn to implement multiple values using Array
Learn object oriented programming using Object and Class
Learn ES6 features - Module, let, const, Arrow Function and Template String Literals
Learn to work with Numbers, String, Array and Dates
Learn to manipulate HTML and CSS using Document Object Model (DOM)
Learn to implement getting data from network using JSON, XMLHttpRequest and fetch
Learn basics of HTTP protocol and HTTP method types
Learn to implement a beginner level project Tic-Tac-Toe
Related Topics
3706014
udemy ID
12/15/2020
course created date
6/21/2021
course indexed date
ANUBHAV JAIN
course submited by