Software Developer - C#/OOP/ASP.NET MVC/MS SQL/SQL Server
Build Applications with C#/OOP/ASP.NET MVC/MS SQL/SQL Server
4.37 (153 reviews)
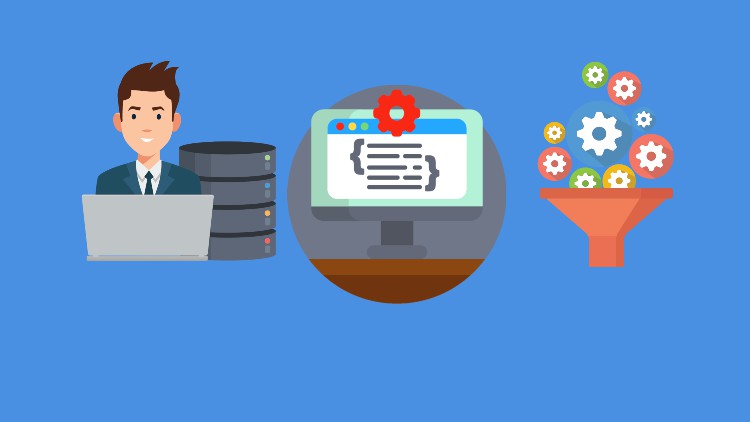
14,744
students
12 hours
content
Dec 2023
last update
$54.99
regular price
What you will learn
Create Console Apps
Create Windows Forms Apps
Create ASP DOT NET Core App
Create your own classes
Learn Object Oriented Programming Concepts with C#
Learn C# Fundamentals
Create database driven apps
Learn MS SQL
Setup SQL Server
Setup Visual Studio
Create ASP DOT NET MVC APP
Related Topics
4344302
udemy ID
10/11/2021
course created date
10/13/2021
course indexed date
Angelcrc Seven
course submited by