Hands-On Data Structures
Hands on Implementation / Coding with Data Structures
4.53 (20 reviews)
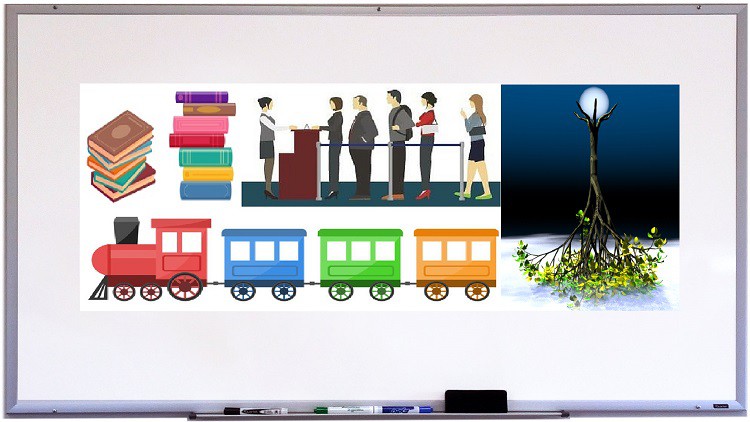
8,903
students
34 hours
content
Aug 2024
last update
$19.99
regular price
What you will learn
Data Structures programming,
Screenshots
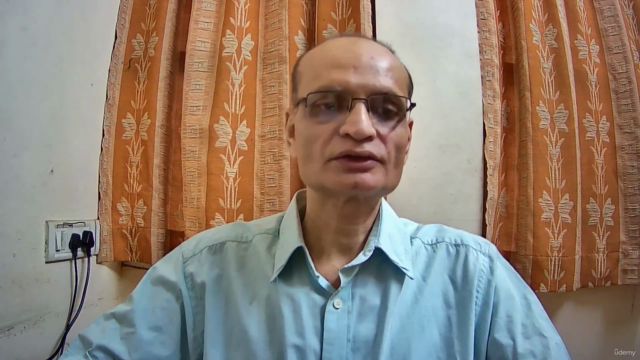
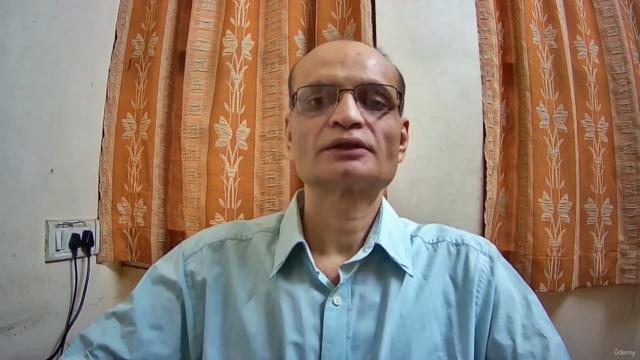
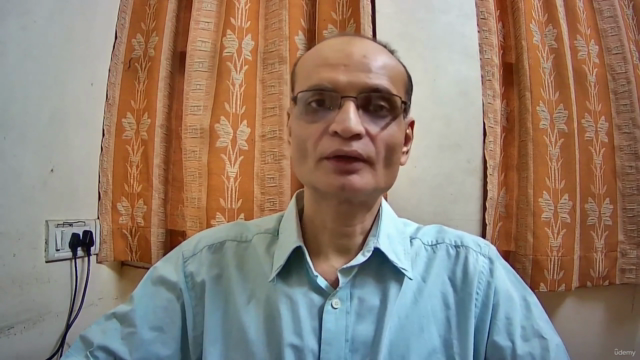
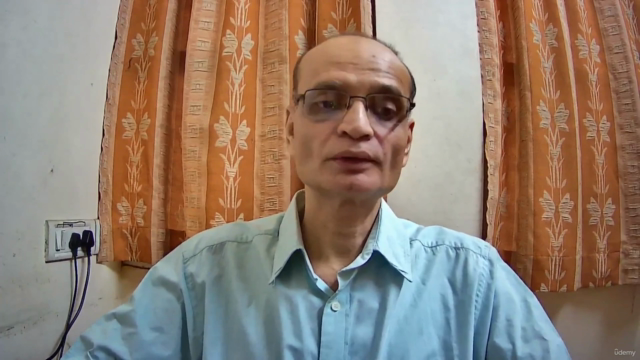
Related Topics
4125068
udemy ID
6/15/2021
course created date
6/20/2021
course indexed date
Bot
course submited by