Using TypeScript with React
Learn how to use TypeScript to build React projects (including Next.js and Apollo GraphQL).
4.42 (1962 reviews)
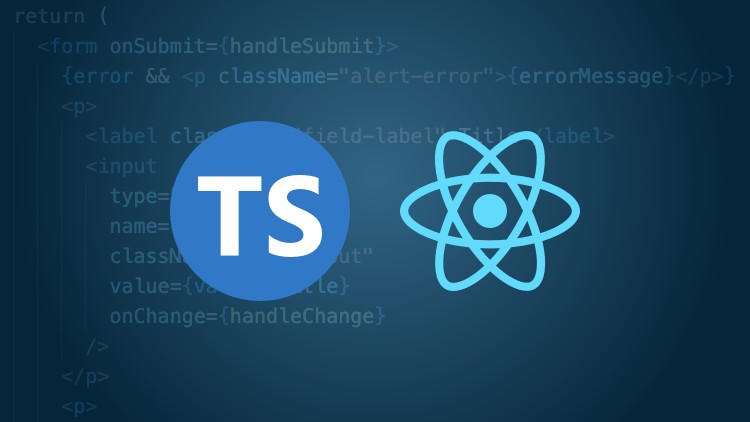
13,277
students
13.5 hours
content
Nov 2024
last update
$84.99
regular price
What you will learn
Basic and advanced features of TypeScript
Using TypeScript in React projects
Writing types for React patterns (higher order components, render props, etc)
How to integrate TypeScript into a Create React App with Redux
How to build a NextJS web app which uses the GraphQL API using TypeScript
Using types provided by third-party packages and creating custom type definitions
Using React with TypeScript in general
Screenshots
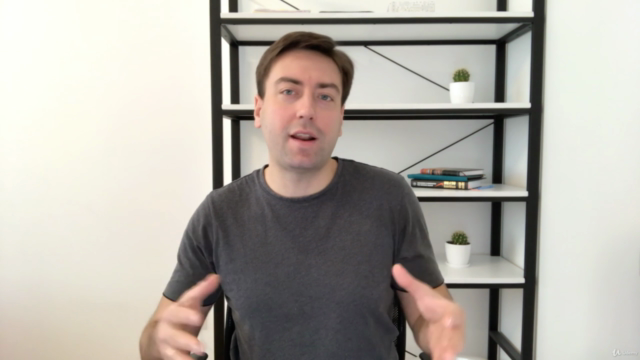
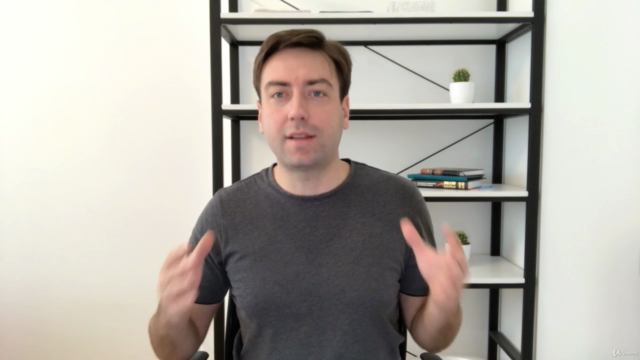
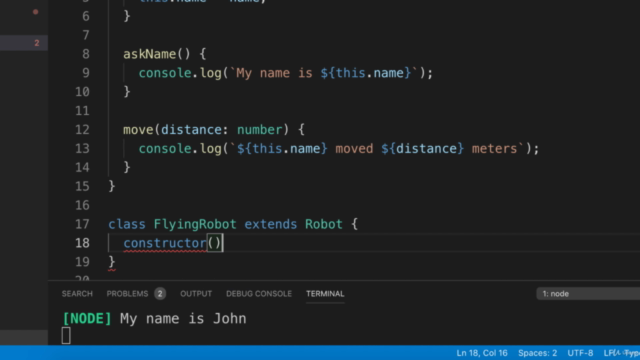
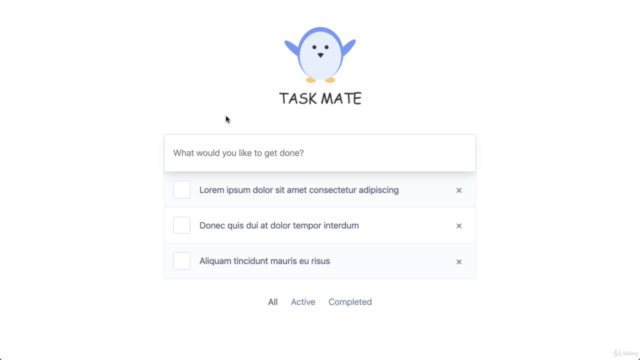
Related Topics
2321154
udemy ID
4/13/2019
course created date
5/10/2019
course indexed date
Bot
course submited by