Complete React, Next.js & TypeScript Projects Course 2025
Hands-on React: 25+ Projects Featuring Next.js, TypeScript, Prisma, Zod, Shadcn, Axios, Router 6, Query 5, Redux Toolkit
4.66 (11837 reviews)
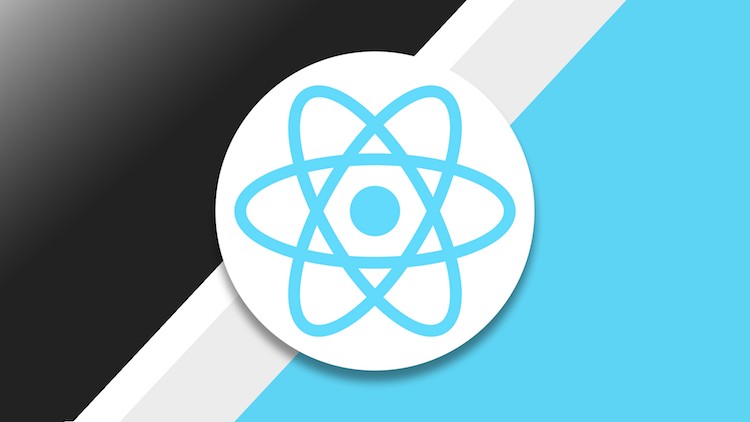
74,902
students
100.5 hours
content
Jan 2025
last update
$109.99
regular price
What you will learn
Make Great Projects Using React
Screenshots
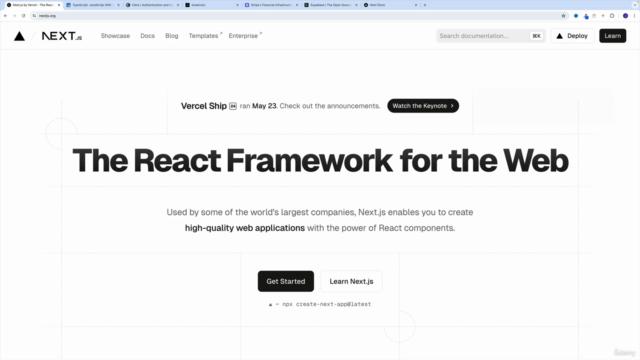
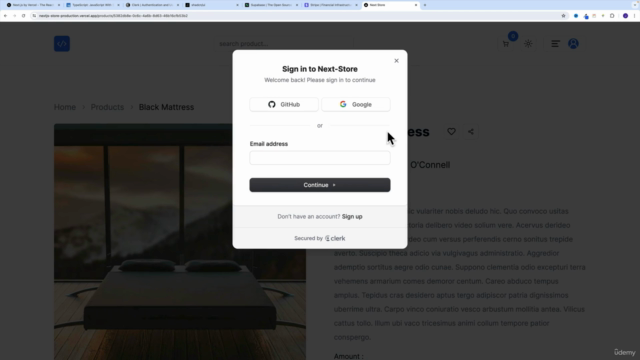
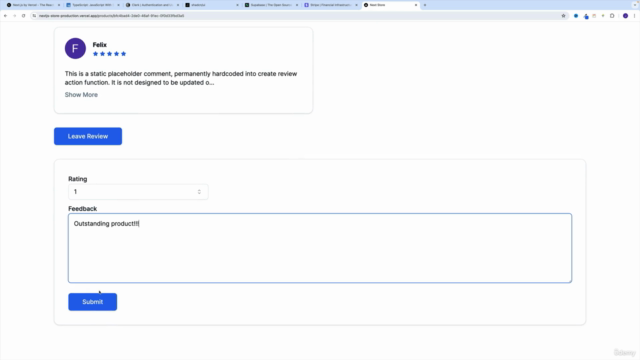
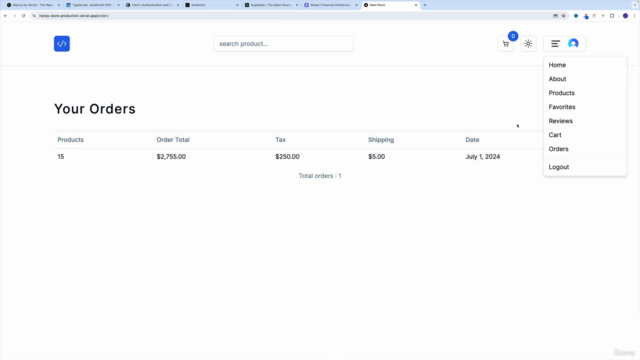
Related Topics
2018828
udemy ID
11/10/2018
course created date
5/13/2019
course indexed date
Bot
course submited by