Object-Oriented Programming for beginners: Python, C++, C#
Learn Object Oriented Programming with Python, C++, C#
4.52 (23 reviews)
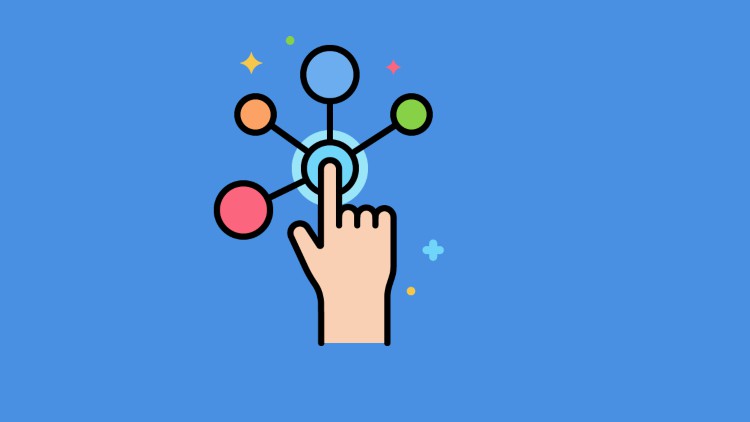
3,347
students
6 hours
content
Aug 2022
last update
$49.99
regular price
What you will learn
Creating Classes and methods
Instantiating
Inheritance
Polymorphism
Encapsulation
Abstraction
Related Topics
4377962
udemy ID
11/2/2021
course created date
11/4/2021
course indexed date
Bot
course submited by