React Node MERN Stack from Scratch building Social Network
Master MERN Stack from Scratch while building awesome Social Network. Covers entire Front/Backend Database & Deployment.
4.33 (1027 reviews)
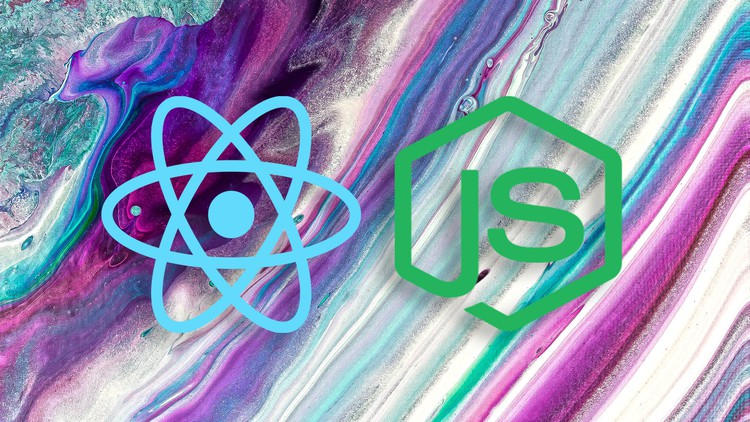
9,595
students
46 hours
content
Feb 2024
last update
$69.99
regular price
What you will learn
Understand Modern JavaScript
Understand Node Js from Scratch
Understand React Js from Scratch
Learn Node JS API Development from Scratch
Learn Frontend Web Development with React
Understand JavaScript in the Browser Environment
Understand JavaScript in the Node JS Environment
How Node JS Event Loop works
Synchronous vs Asynchronous programming
Blocking vs Non-Blocking code
Using Core Node JS Modules
Using your own Modules
Using NPM Modules
MVC Design Pattern
Learn Mongo DB
Learn to implement CRUD (create, read, update, delete) on users/posts
Learn to use Postman
Implement Authentication using JWT
Implement Social Login
Password Forgot/Reset Functionality
User Post Relationship
Authorization and Permissions
Admin Role/Dashboard
User Profile
Image Upload
User Follow/Unfollow
Post Like/Unlike
Comments
Deploy Node JS API to Digital Ocean Cloud Server
Deploy React JS Web App to Digital Ocean Cloud Server
Custom ReCaptcha
Pagination
Build A Complete Social Network Application
Real Time with SocketIo
SEO (Server Side Rendering) with NextJs
Screenshots
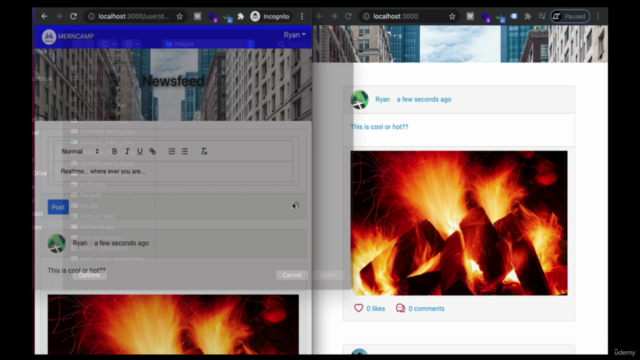
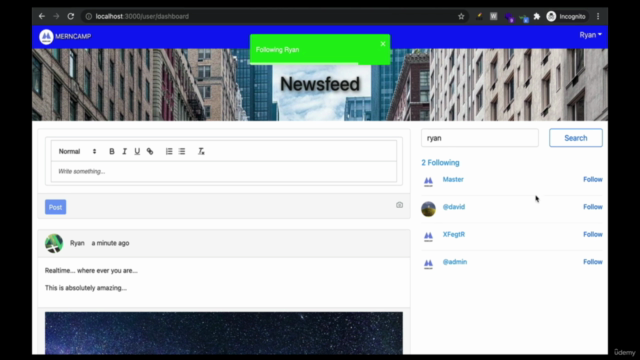
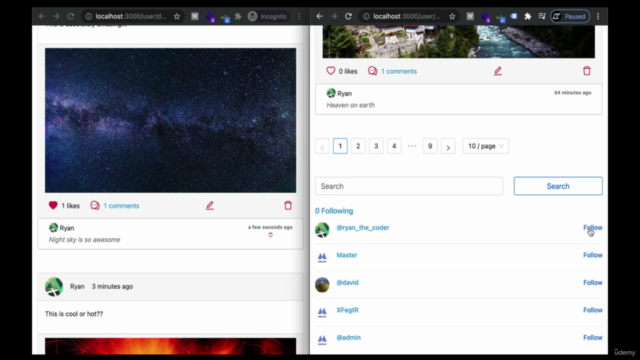
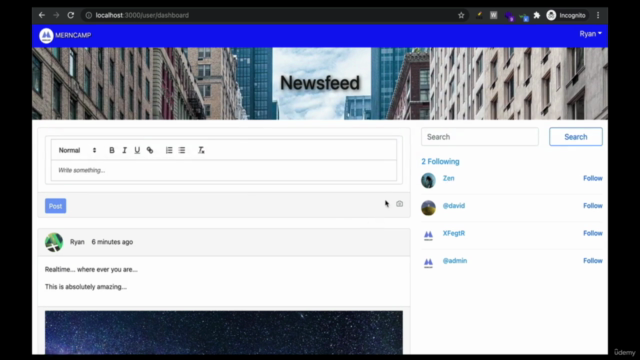
2143416
udemy ID
1/11/2019
course created date
3/12/2019
course indexed date
Bot
course submited by