NFT Marketplace - React, Next.js & Solidity Guide (2023)
Build Web3 NFT Marketplace on Ethereum using React/Next, Solidity, and Pinata (IPFS), with Typescript. Covers Polygon.
4.46 (234 reviews)
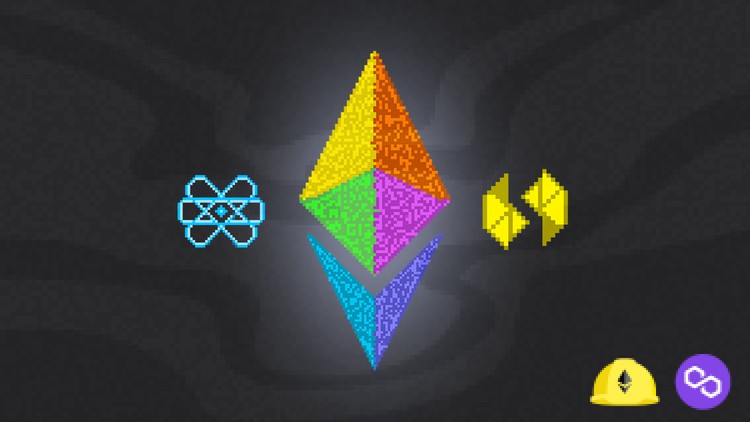
2,572
students
22.5 hours
content
Dec 2023
last update
$84.99
regular price
What you will learn
Build an authentic NFT marketplace
Understand complex topics in practical and fun way
Get complete toolkit to work with any Blockchain
Develop a real app on the Blockchain
Screenshots
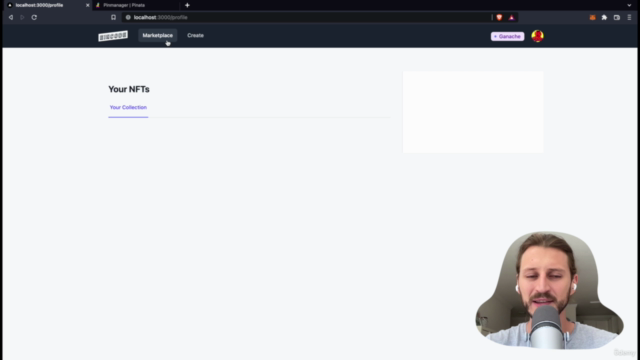
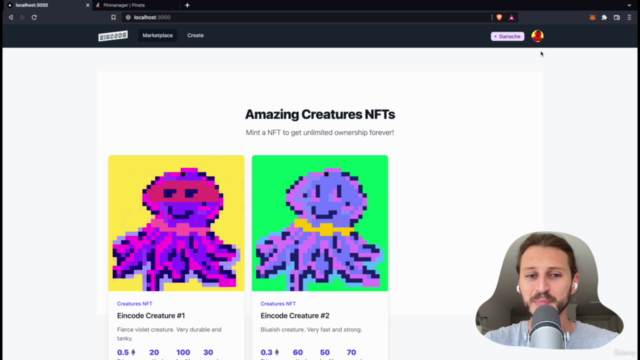
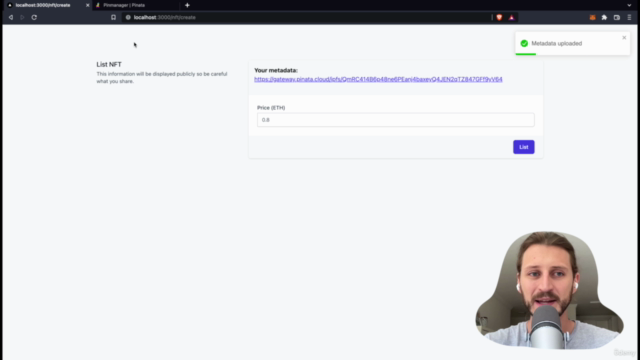
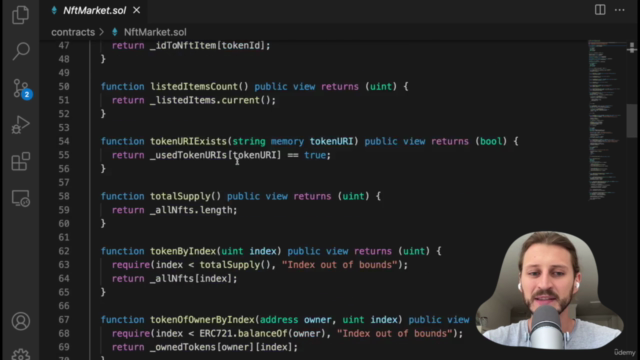
Related Topics
4634138
udemy ID
4/9/2022
course created date
5/10/2022
course indexed date
Bot
course submited by