Advanced JavaScript Topics
In Depth JavaScript Training for Mastering Important Patterns, the Power of Functions, OOP Concepts, JavaScript Projects
4.39 (842 reviews)
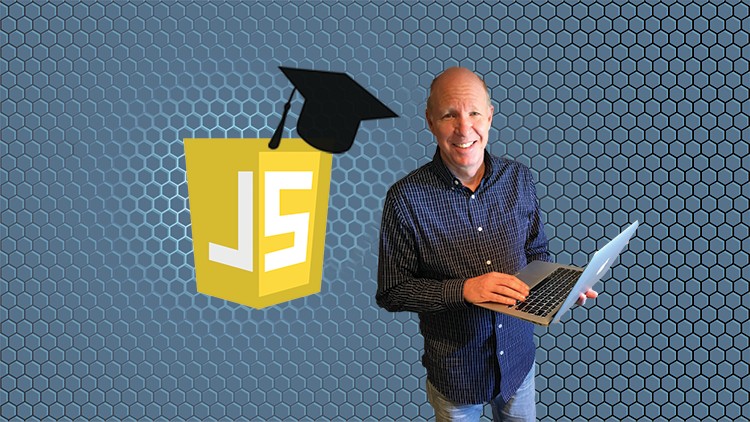
7,145
students
19 hours
content
Jul 2024
last update
$84.99
regular price
What you will learn
Apply first-class and higher order functions in your coding practices.
Explain the different scenarios that affect the value of this.
Identify the object to which this is bound.
Manipulate this binding to accomplish programing problems.
Use prototypes in your coding.
Understand and use IIFEs in your code.
Define closure and take advantage of it in your code.
Apply the namespace and module pattern to your coding projects.
Create JSON files.
Load and use JSON data in a project.
Manipulate properties on JavaScript objects.
Apply OOP principles to your JavaScript coding practices.
Make use of constructors and Object create for setting up objects and prototypes.
Understand and apply the true nature of JavaScript inheritance.
Explain functional programming concepts.
Apply functional programming techniques to your JavaScript projects.
Screenshots
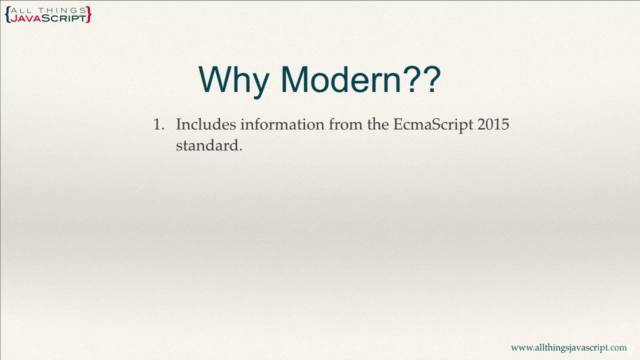
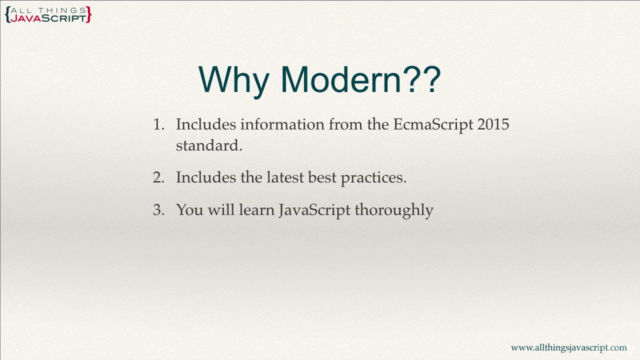
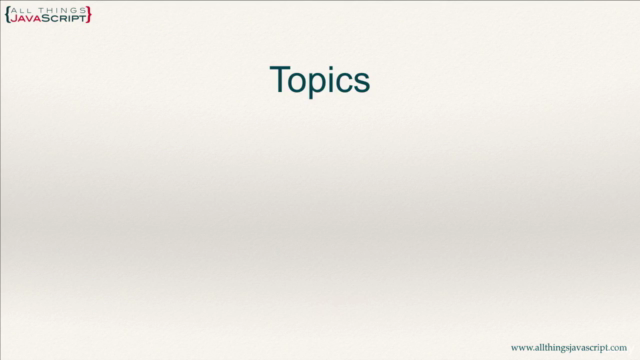
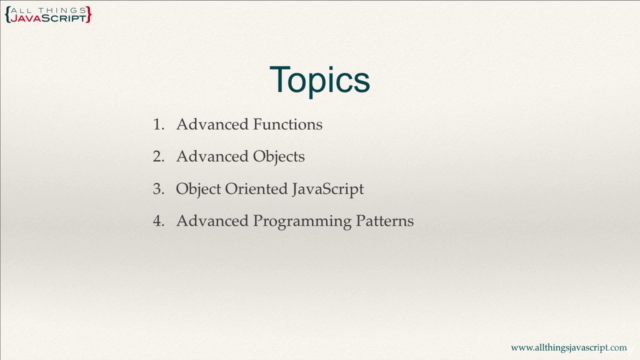
Related Topics
1072420
udemy ID
1/11/2017
course created date
4/27/2019
course indexed date
Bot
course submited by