Java Fundamentals: Your Guide to Modern Software Development
Master the core concepts of Java programming language and apply them to practical projects. Become a top Java Developer.
4.25 (319 reviews)
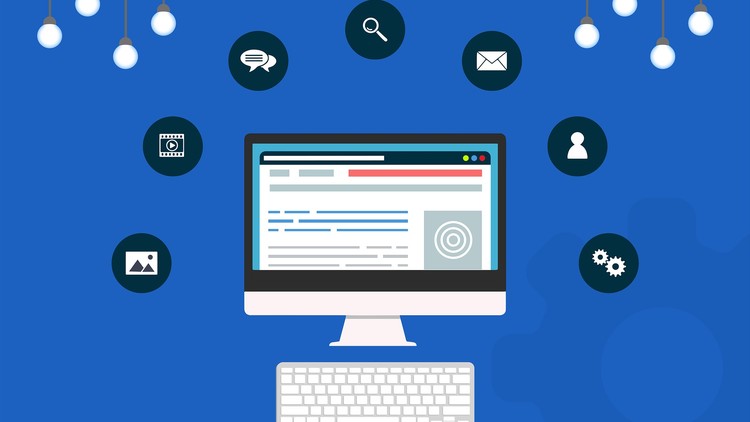
23,704
students
23 hours
content
Jul 2024
last update
$19.99
regular price
What you will learn
Get end-to-end understanding of core concepts of Java
Learn the core Java skills needed to apply for Java developer positions
Master design principles, best practices and coding conventions for writing well-designed, professional Java code
Be able to demonstrate your understanding of Java to future employers
Prepare and apply for Oracle Java Certification exams (Oracle Certified Associate, Java SE 8 Programmer 1Z0-808)
Understand Object Oriented Programming concepts like as classes and objects, threads, files, applets, swings, and act are essential
Master OOP fundamentals and use real-world applications as case study
Learn why Java is useful for the design of desktop and web applications
Identify Java language components and how they work together in applications
Design and program stand-alone Java applications
How to design a graphical user interface (GUI) with Java Swing
Learn how to extend Java classes with inheritance and dynamic binding
Use exception handling in Java applications
Understand how to design GUI components with the Java Swing API
Learn Java generics and how to use the Java Collections API
Write Programs using the Java Graphical User Interface
Understand how to design applications with threads in Java
Analyze event-driven programming techniques, such as generating and manipulating objects and classes, and utilizing Java for network programming and middleware
Learn how to read and write files in Java
Learn multi-tier applications and learn to design and develop them
Analyze and identify enterprise applications
Create a Java application for a distributed system
Evaluate Java programming for networking ideas includes writing, compiling, executing, and troubleshooting
Screenshots
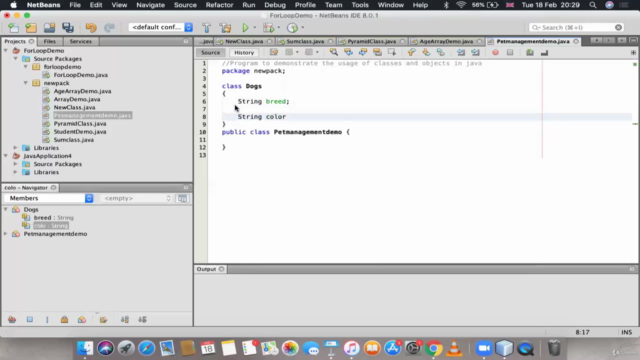
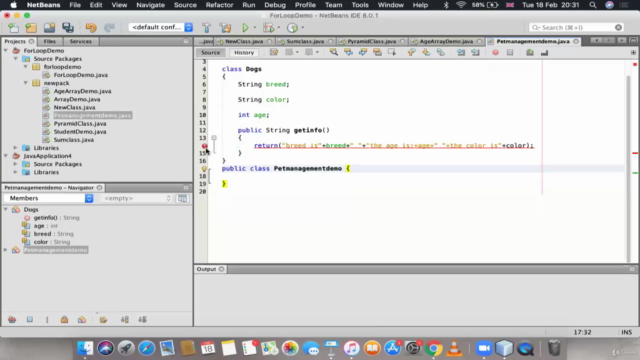
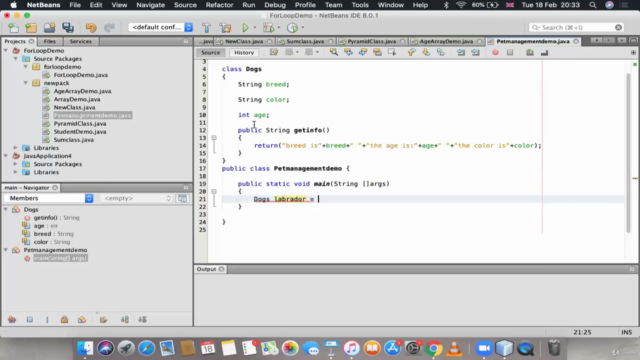
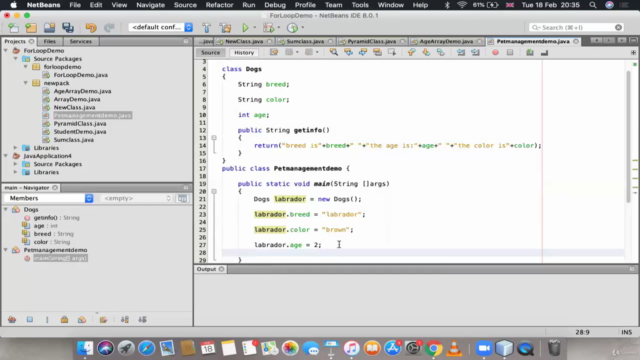
Related Topics
2830346
udemy ID
2/22/2020
course created date
5/8/2020
course indexed date
Bot
course submited by