Title
Java Concurrency & Multithreading: beginner to intermediate
Learn Java multi-threading and resolve data and race conditions with volatile, atomics, and synchronized
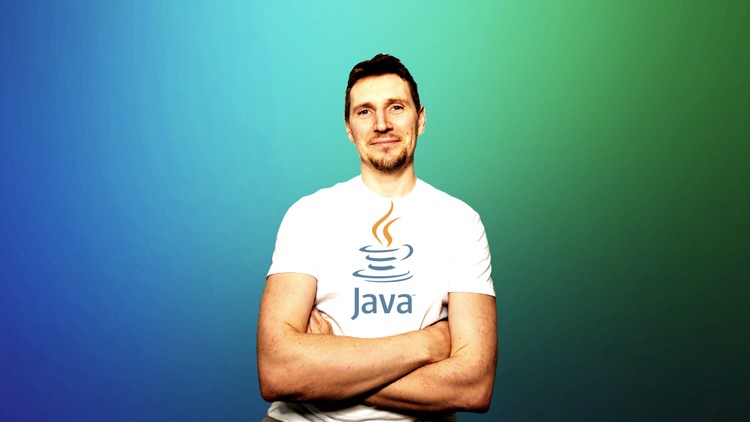
What you will learn
A high-level overview of process management by the operating system
A high-level overview of thread management by the operating system
The fundamental difference between a process and a thread
How to define a thread using the Thread subclassing approach
How to define a thread by implementing the Runnable interface
The different states of a thread and its lifecycle
How to start threads using the start() method and the ExecutorService
The strategy for reusing threads employed by the ExecutorService
How to define a thread by implementing the Callable interface
How to use the Future interface in blocking and non-blocking ways
The usage of daemon threads
The functionality of the Thread's .join() and .interrupt() methods
The concept of data race and its details
How to use the 'volatile' keyword to avoid data race
The concept of mutex / intrinsic lock / monitor
The concept of race condition, its details and difference from the data race
How to use the 'synchronized' keyword to implement mutex/monitor acquire in Java
The concept of the compare-and-swap CPU instruction
A high-level overview of the Java atomics package and its relation to compare-and-swap
How to use the AtomicInteger class to avoid arithmetic operation race condition
The usage of the ThreadLocal class in Java
How to take thread dumps of the JVM process
How to use the .parallelStream() method in the Java Streaming API
Why take this course?
🎓 Java Concurrency & Multithreading: Beginner to Intermediate
🚀 Course Headline: Unlock the full potential of your Java applications by mastering concurrency and multithreading! Dive deep into the world of parallel computing, resolve data races, and optimize your application performance.
🔍 Course Description: In today's fast-paced world, writing efficient, responsive, and scalable Java applications is essential. This course is designed to take you from a beginner to an intermediate level in understanding and implementing concurrency and multithreading concepts in Java. You'll learn the theoretical underpinnings alongside practical coding examples that leverage the robust functionalities provided by the Java Development Kit (JDK).
What You'll Learn:
-
The Fundamentals of Processes & Threads: Gain a solid foundation on how operating systems handle processes and threads, and understand their differences.
-
Creating and Managing Threads in Java:
- Learn to define threads using
Thread
,Runnable
, andCallable
. - Explore the various states a thread can be in and the stages of its lifecycle.
- Learn to define threads using
-
Advanced Thread Management:
- Discover multiple methods for starting new threads, including
ExecutorService
and parallel streams. - Get to grips with the ExecutorService thread pool reuse strategies.
- Discover multiple methods for starting new threads, including
-
Working with Futures and Thread Interruptions:
- Understand blocking and non-blocking operations when working with futures.
- Use the
.join()
method to wait for a thread's completion and learn how to use.interrupt()
to handle interruptions properly.
-
Daemon Threads Explained:
- Learn what daemon threads are, why they exist, and how they differ from user threads.
-
Concurrency Mechanisms:
- Understand data races and the importance of atomic operations to mitigate them using the
volatile
keyword. - Grasp the concept of race conditions and how to resolve them with mutex strategies.
- Understand data races and the importance of atomic operations to mitigate them using the
-
Synchronization in Java:
- Write Java code that implements synchronization, solving concurrency issues.
- Dive into the use of the synchronized keyword for mutual exclusion.
-
Java Atomic Packages:
- Explore the
atomic
package and its uses, particularly focusing onAtomicInteger
. - Understand the compare-and-swap instruction and how it's used within Java.
- Explore the
-
Advanced Concurrency Tools:
- Familiarize yourself with the
ThreadLocal
class for thread-specific data. - Learn how to analyze your application with thread dumps, diagnosing issues in real-time.
- Familiarize yourself with the
By the end of this course, you'll have a comprehensive understanding of Java concurrency and multithreading, enabling you to write efficient and robust applications that can handle complex tasks with ease. Whether you're developing high-performance servers or multiplayer games, this knowledge will be invaluable.
Join us on this journey to master one of the most critical aspects of Java programming! 🌟
Our review
🌟 Course Review for "Mastering Concurrency & Multithreading in Java" 🌟
Overall Rating: 4.8/5
Course Summary and Pros:
- Target Audience: Ideal for beginners looking to grasp the fundamentals of concurrency and multithreading in Java. Also beneficial for experienced programmers seeking to solidify or expand their knowledge.
- Content Structure: The course is well-structured, with information presented in a clear and easy-to-understand manner.
- Instructional Approach: The instructor's expertise shines through with every explanation being conveyed with clarity and precision.
- Real-Life Examples: The use of concrete examples, such as the bakery analogy and scenarios involving cashiers like "Alice" and "Bob," make complex concepts more accessible.
- Practical Application: The course provides practical Java examples that enhance learning and application of the concepts taught.
- Recommendation: Highly recommended for anyone interested in deepening their understanding of Java concurrency and multithreading. The course's educational approach is engaging and effective, as evidenced by numerous positive reviews.
Areas of Improvement:
- Examples Needed: Some reviewers suggest that the course could benefit from a wider variety of examples beyond the ones currently provided, including more concrete scenarios to illustrate concepts.
- Depth of Examples: A few users recommend that the real-life examples should be further elaborated upon to provide a more in-depth understanding and application of concurrency and multithreading principles.
Key Takeaways from Reviews:
- The course is considered "perfect for beginners" and is commended for its well-explained content.
- Reviewers appreciate the clear, simple explanations and the structured presentation of information.
- The instructor's ability to make complex topics easy to understand is a standout feature of this course.
- The examples used in the course, including the bakery example, are praised for their effectiveness in explaining concurrency concepts.
- The course content is said to provide a solid foundation and is recommended as the best on Java multithreading available on Udemy.
In conclusion, this course is highly regarded by users who have found it not only informative but also engaging and beneficial for both beginners and experienced Java developers. The feedback indicates that while the course excels in many areas, there is a slight opportunity for improvement in the breadth and depth of real-life examples to further enhance the learning experience.
Charts
Price

Rating

Enrollment distribution
