How to Program Games: Tile Classics in JS for HTML5 Canvas
Learn to code tile-based worlds and related core gameplay for genres like arcade, overhead racing, and puzzle adventure.
4.49 (2020 reviews)
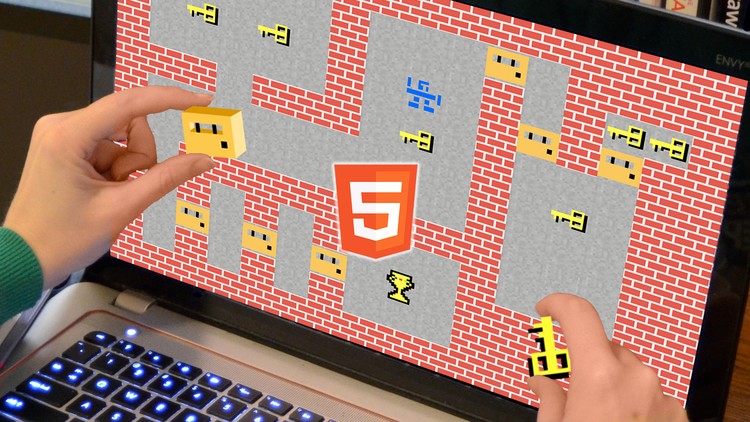
10,280
students
14.5 hours
content
Jul 2020
last update
$109.99
regular price
What you will learn
Create, display, and play with a 2D tile world that supports optimized collision (a central concept for generations of games in a variety of genres!)
Program games in JavaScript for HTML5 Canvas without using any external libraries or plug-ins
Create, load, display, and rotate image graphics in games
Break game code into multiple files to better manage large projects
Define a class and use it to create multiple instances of gameplay objects in unique positions (note: only using the very basic first concept of object-oriented programming, it doesn't dive deep into that rabbit hole)
Handle mouse input for a one-player game, or keyboard controls for both one and two-player games
Implement basic item pick-ups (keys) and trigger their usage upon collision (open doors)
Develop and adapt gameplay for basic platformer movement, digital board/strategy games, simple matrix formations for retro arcade-style enemies, and worlds larger than the screen viewed by scrolling camera
Apply simple trigonometry calls to move game objects at arbitrary angles
Implement basic loading screen functionality in HTML5
Screenshots
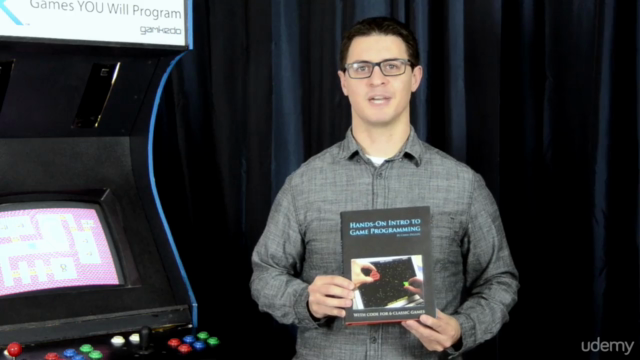
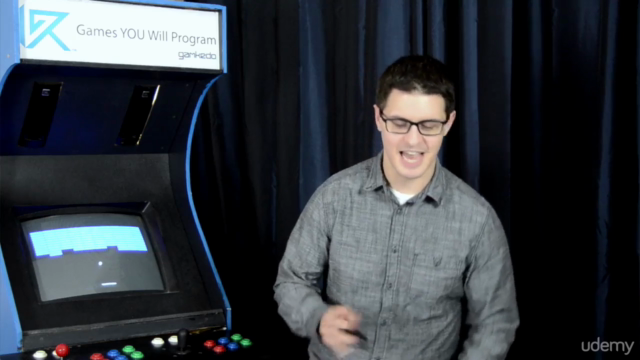
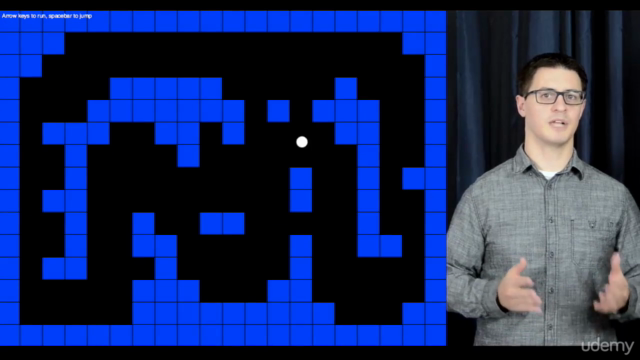
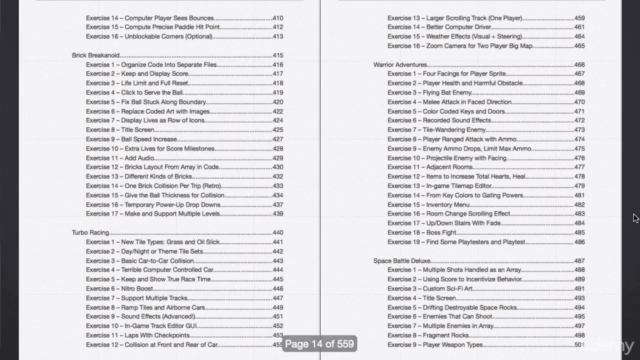
535562
udemy ID
6/23/2015
course created date
11/16/2019
course indexed date
Bot
course submited by