Go: The Complete Developer's Guide (Golang)
Master the fundamentals and advanced features of the Go Programming Language (Golang)
4.61 (39927 reviews)
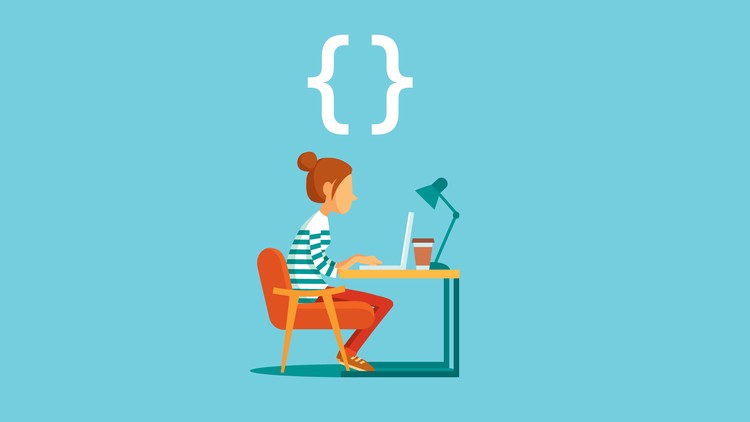
185,773
students
9 hours
content
Apr 2025
last update
$99.99
regular price
What you will learn
Build massively concurrent programs with Go Routines and Channels
Learn the advanced features of Go
Understand the differences between commonly used data structures
Prove your knowledge with dozens of included quiz questions
Apply Interfaces to dramatically simplify complex programs
Use types to future-proof your code and reduce the difficulty of refactors
Screenshots
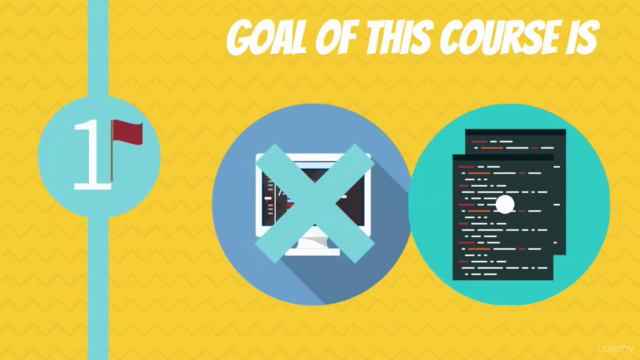
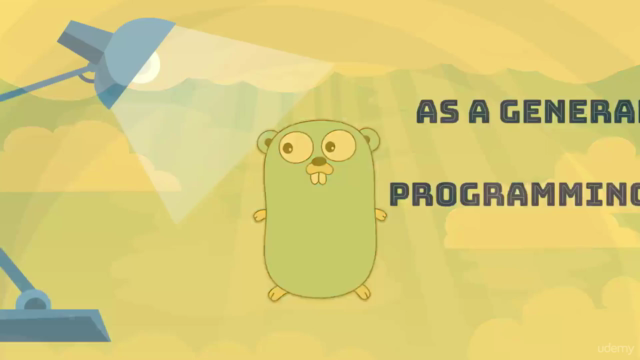
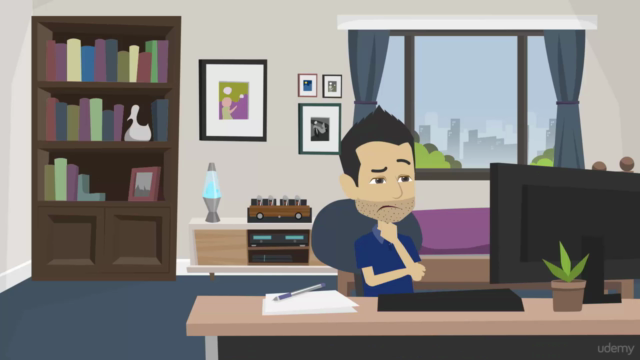
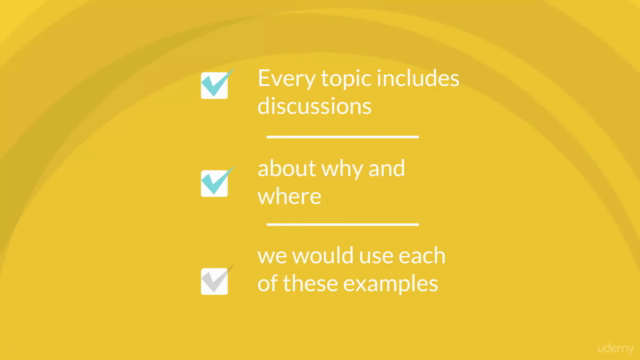
Related Topics
1309202
udemy ID
7/31/2017
course created date
11/20/2019
course indexed date
Bot
course submited by