Fundamentals of Object Oriented Programming with C++
Learn C++ syntax basics and modern Object-Oriented Programming techniques and tips
4.79 (14 reviews)
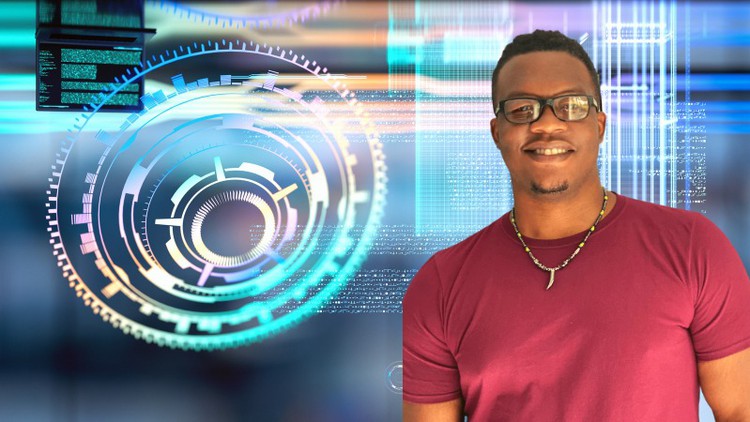
3,178
students
7 hours
content
Jan 2023
last update
$54.99
regular price
What you will learn
Understand the purpose of OOP, the history of the C++ programming language, the anatomy of a C++ program and the role of the compiler
Learn how to use C++ syntax to store, process and retrieve data, take input from a user and provide an output by writing simple programs
Use the built-in STD & STL libraries to process input and data, create custom libraries, file handling and defensive programming techniques
Construct complex - real world object-oriented classes and solutions in C++ based on UML diagrams.
Learn core programming concepts that will improve logical reasoning, critical thinking & problem solving skills and equip you for your first Jr Developer Role
Related Topics
4360140
udemy ID
10/21/2021
course created date
1/1/2023
course indexed date
Bot
course submited by