From 0 to 1: Learn Java Programming -Live Free,Learn To Code
An accessible yet serious guide to Java programming for everyone
4.08 (222 reviews)
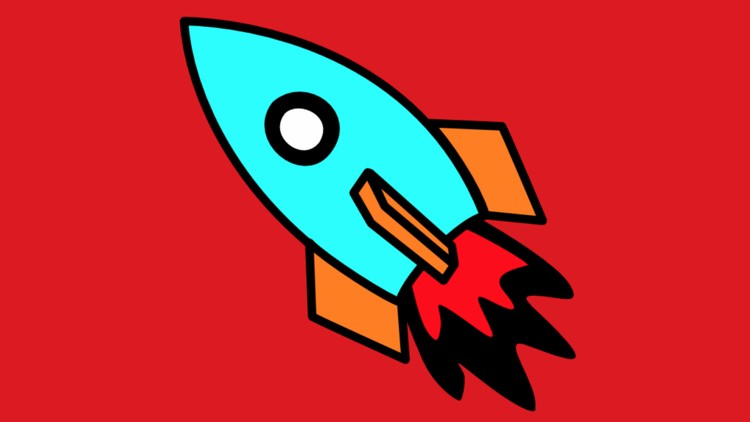
4,734
students
17 hours
content
Feb 2016
last update
$54.99
regular price
What you will learn
Write Java programs of moderate complexity and sophistication (at an early to middling intermediate level)
Understand Object-Oriented programming concepts at the level where you can have intelligent design conversations with an experienced software engineer
Manage concurrency and threading issues in a multi-threaded environment
Create and modify files (including Excel spreadsheets) and download content from the internet using Java
Use Reflection, Annotations, Lambda functions and other modern Java language features
Build serious UI applications in Swing
Understand the Model-View-Controller paradigm, the Observer and Command Design patterns that are at the heart of modern UI programming
Gain a superficial understanding of JavaFX and Properties and Bindings
Understand the nuances of Java specific constructs in serialisation, exception-handling, cloning, the immutability of strings, primitive and object reference types
Screenshots
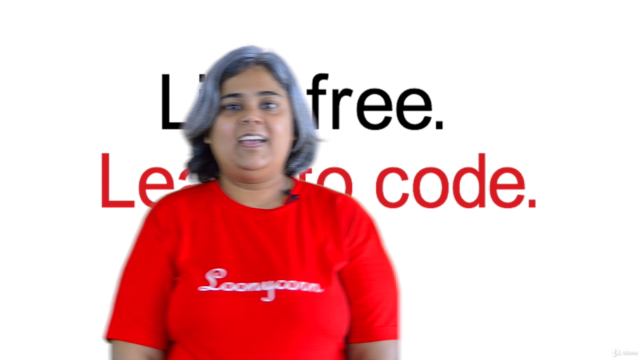
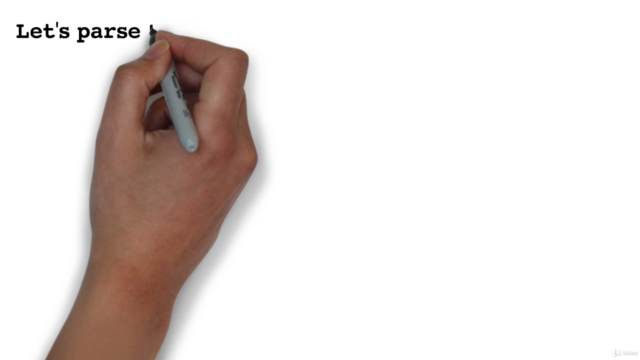
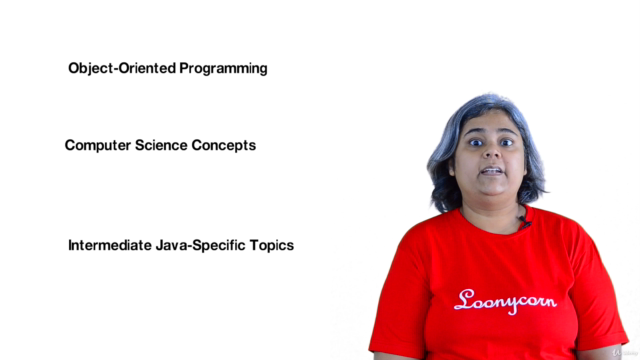
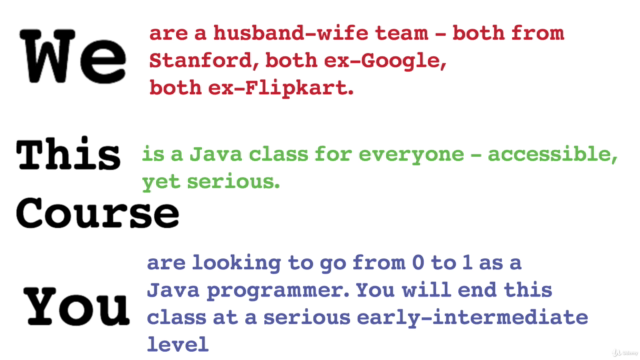
Related Topics
639048
udemy ID
10/13/2015
course created date
12/9/2020
course indexed date
lelos
course submited by