Building an Interpreter from scratch
Semantics of programming languages
4.84 (279 reviews)
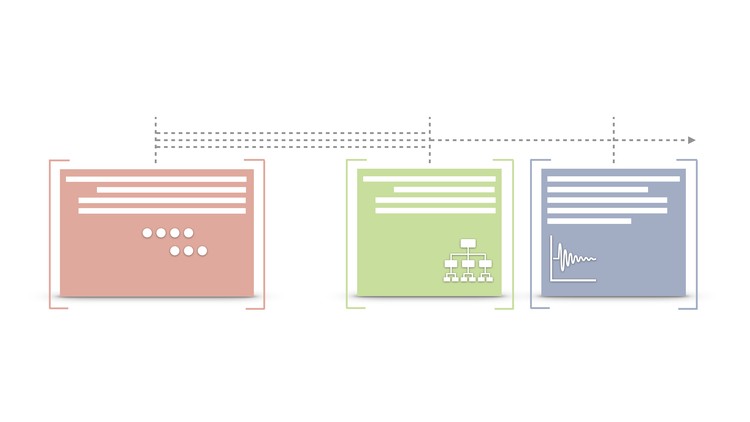
1,189
students
3 hours
content
Sep 2020
last update
$49.99
regular price
What you will learn
Build a programing language from scratch
Interpreters and Compilers
AOT, JIT-compilers and Transpilers
AST-interpreters and Virtual Machines
Bytecode, LLVM, Stack-machines
First-class functions, Lambdas and Closures
Call-stack and Activation Records
OOP: Classes, Instances and Prototypes
Modules and Abstractions
Related Topics
2845910
udemy ID
3/2/2020
course created date
3/21/2020
course indexed date
Bot
course submited by