Build a Backend REST API with Python & Django - Advanced
Create an advanced REST API with Python, Django REST Framework and Docker using Test Driven Development (TDD)
4.63 (9318 reviews)
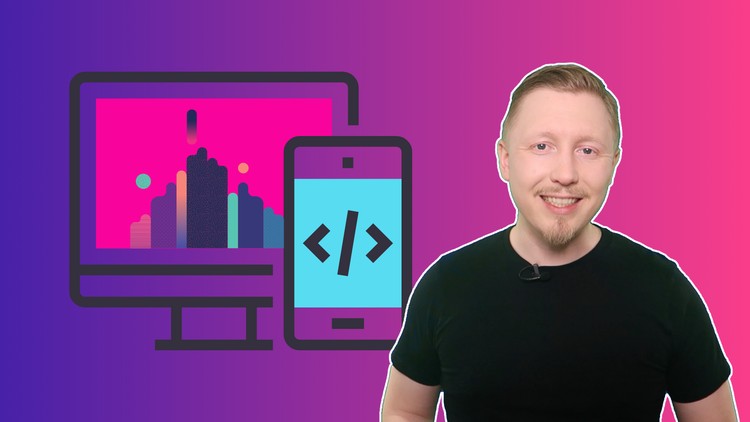
52,838
students
15 hours
content
Aug 2024
last update
$149.99
regular price
What you will learn
Setting up a local development server with Docker
Writing a Python project using Test Driven Development
Building a REST API with advanced features such as uploading and viewing images
Creating a backend that can be used a base for your future projects or MVP
Hands on experience applying best practice principles such as PEP-8 and unit tests
Configure Travis-CI to automate code checks
Screenshots
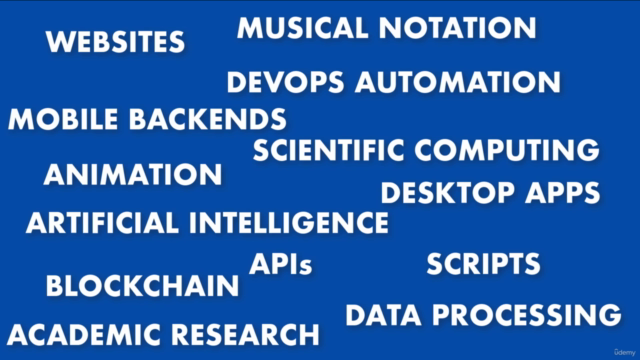
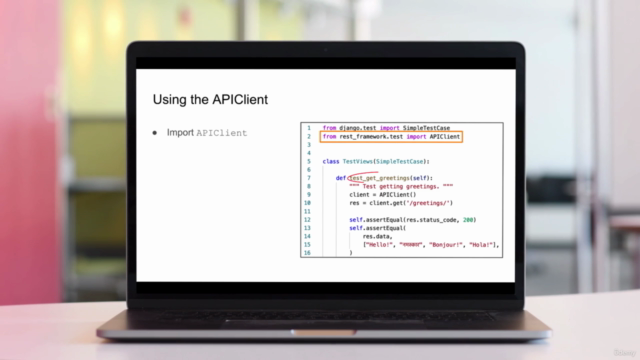
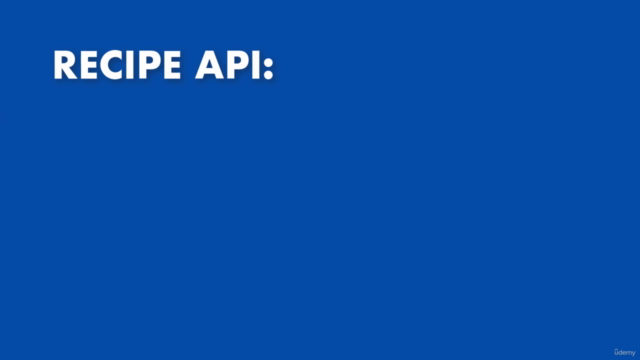
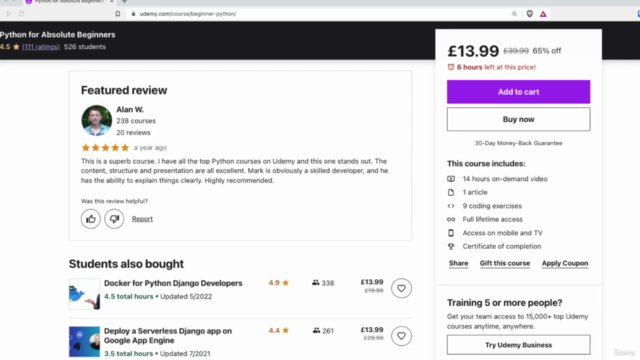
Related Topics
2045310
udemy ID
11/24/2018
course created date
8/10/2019
course indexed date
Bot
course submited by