Django Database ORM Mastery
Learn how to master building and interacting with databases within a Django project
4.37 (437 reviews)
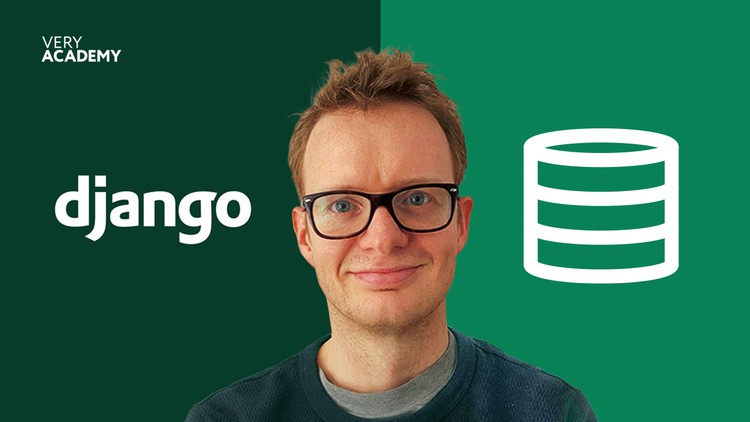
3,979
students
49.5 hours
content
Mar 2025
last update
$84.99
regular price
What you will learn
Setup and prepare an environment ready to develop Python Django applications
Develop Django models, manage models and create Django admin model interactions
Connect a Django application to multiple database technologies utilising Docker container technologies
Propagate changes to database tables structures
Build raw SQL queries to interact with database from within a Django project
Implement a variety of QuerySet API methods to limit, order and optimise performance
Implement a design methodology design and subsequently build and query a database
Related Topics
4721466
udemy ID
6/6/2022
course created date
10/8/2022
course indexed date
Bot
course submited by