GUI Development with Python and Tkinter
Master Python GUI development using Tkinter to build desktop applications!
4.75 (3089 reviews)
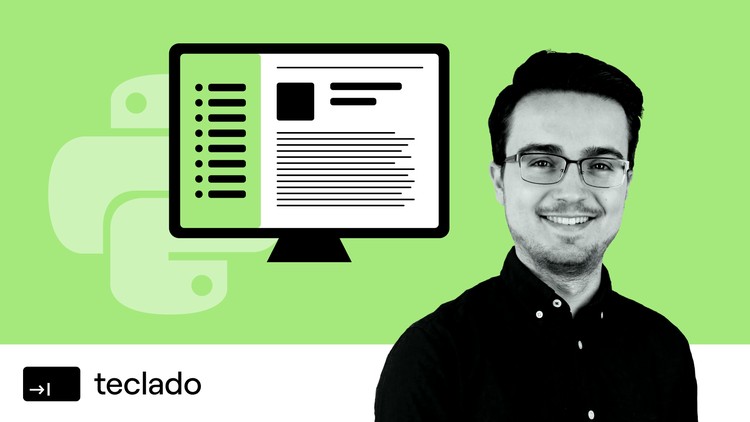
19,376
students
11.5 hours
content
Feb 2025
last update
$99.99
regular price
What you will learn
Master GUI Development with Tkinter and Python!
Create multiple Tkinter projects, including forms, games, and even a chat app that interacts with a web API.
Fully understand the two most important Geometry Managers in Tkinter: grid and pack.
Learn how to use a wide variety of widgets, such as labels, entries, buttons, spinboxes, and even the Canvas!
Gain in-depth knowledge of how themes and styles work in Tkinter, as well as how you can create your own styles and use them in your applications.
Throughout the entire course, follow best practices for Python and Tkinter code as taught by a professional software developer with years of experience.
Related Topics
2589314
udemy ID
10/3/2019
course created date
11/8/2019
course indexed date
Bot
course submited by