Design Patterns in TypeScript
Write cleaner code and enhance your development skills with TypeScript design patterns
4.02 (195 reviews)
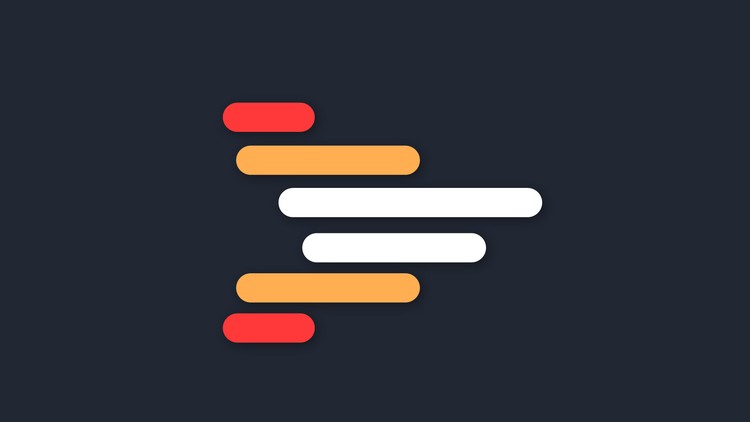
1,242
students
5 hours
content
Sep 2018
last update
$49.99
regular price
What you will learn
Identify and avoid violations of the SOLID Principles
Create single instances of classes throughout your apps with the Singleton pattern
Use factories to get instances and object pools
Understand Dependency Injection and work with its benefits
Use InversifyJS and learn about dependency scope
Extend objects with the Decorator pattern
Convert interfaces using the Adapter and Facade patterns
Choose implementations on runtime using the Strategy pattern
Use the Observer pattern to notify your app's components about changes
Store application state using the State pattern
Screenshots
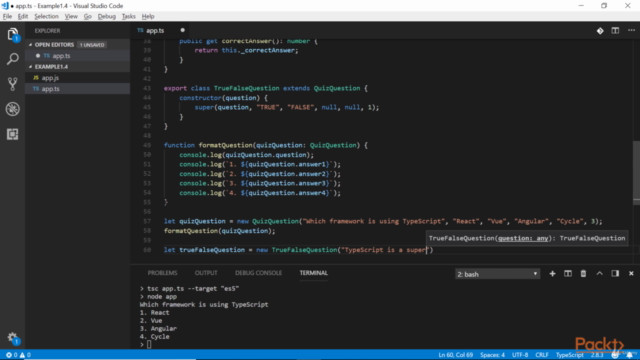
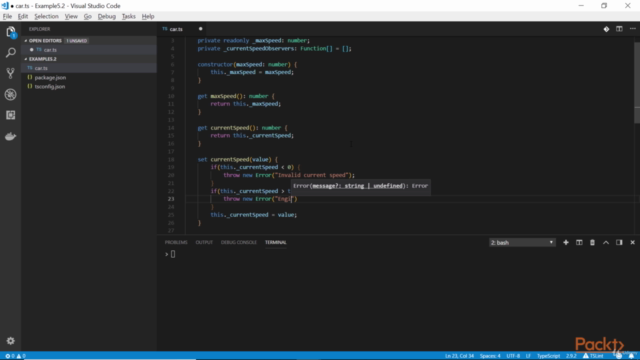
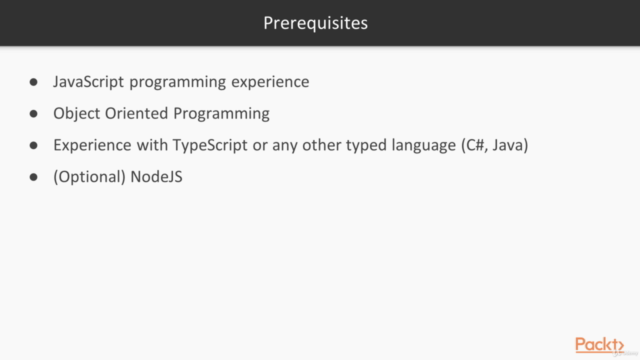
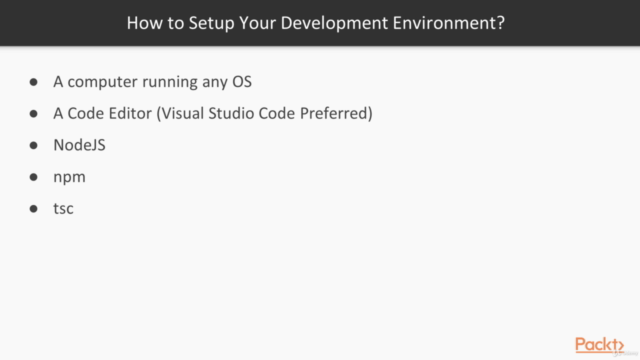
Related Topics
1897768
udemy ID
9/6/2018
course created date
8/2/2019
course indexed date
Bot
course submited by