Fundamental Data Structures & Algorithms using C language.
Learn Data Structures and algorithms for Stack, Queue, Linked List, Binary Search Tree and Heap ( using C Programming ).
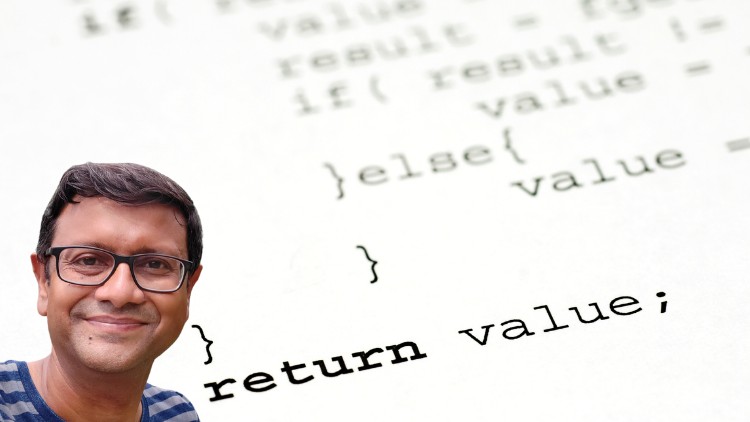
What you will learn
Recursion, Stack, Polish Notations, infix postfix, FIFO, Circular & Double Ended Queue, Linked List - Linear, Double & Circular, Stack & Queue using Linked List
What is stack, algorithms for Push and Pop operation. Implementation of Stack data structure using C.
Using Stack - checking parenthesis in an expression
Using Stack - Understanding Polish notations, algorithm and implementation of infix to postfix conversion and evaluation of postfix expression
What is a FIFO Queue, understanding Queue operations - Insert and delete, implementing FIFO Queue
Limitations of FIFO queue, concept of Circular Queue - Implementation of Circular queue.
Concept of Double ended queue, logic development and implementation of double ended queue.
Concept of Linked List - definition, why we need linked list.
Singly Linked List - developing algorithms for various methods and then implementing them using C programming
Doubly Linked List - developing algorithm of various methods and then implementing them using C programming
Circular Linked List - developing algorithm of various methods and then implementing them using C programming
How to estimate time complexity of any algorithm. Big Oh, Big Omega and Big Theta notations.
Recursion, concept of Tail recursion, Recursion Vs Iteration.
Binary Tree, definition, traversal (in-order, pre-order and post-order), binary search tree, implementation.
Heap - concept, definition, almost complete binary tree, insertion into heap, heap adjust, deletion, heapify and heap sort.
Why take this course?
This course will help the students ability to grasp the knowledge of data structures and algorithm using the C programming language. Knowledge of Data Structures and Algorithms are essential in developing better programming skills.
This course is based on the standard curriculum of Universities across the globe for graduate level engineering and computer application course.
Apart from step by step development of concepts students will also learn how to write algorithms and then how to write programs based on the algorithms in this course.
You will learn the following in this course: (All implemented using C programming)
Fundamental of Data Structure concept
Why we need Data Structures
Stack - Idea, definition, algorithm, implementations.
Using Stack - Parenthesis checking, Polish Notation, Infix to postfix conversion and evaluation.
FIFO Queue - Idea, definition, algorithm, implementation.
Circular Queue using array - Idea, definition, algorithm, implementation.
Double ended queue using array - Idea, definition, algorithm, implementation.
Linked List - Idea, definition, why we need linked list. Comparison with array.
Singly Linked List - Development of algorithm for various operations and then Implementation of each of them
Creating Stack and Queue using Singly Linked list - Implementation.
Doubly Linked List - Idea, definition, algorithm of various operations and implementations.
Circular Linked List - Idea, definition, algorithm and implementations.
14. Calculating efficiency of algorithms, Worst Case (Big Oh), Average Case (Big Theta) and Best case (Big omega) complexities. How to calculate them for different algorithms.
15. Binary Searching
16. Recursion in detail. Example program using recursion and the critical comparison between Recursive approach and Iterative approach of problem solving.
17. Binary Tree, definition, traversal (In, Pre and Post Order), Binary Search Tree implementation.
18. Heap data structure, definition, heap insertion, deletion, heap adjust, Heapify and heap sort.
Reviews
Charts
Price
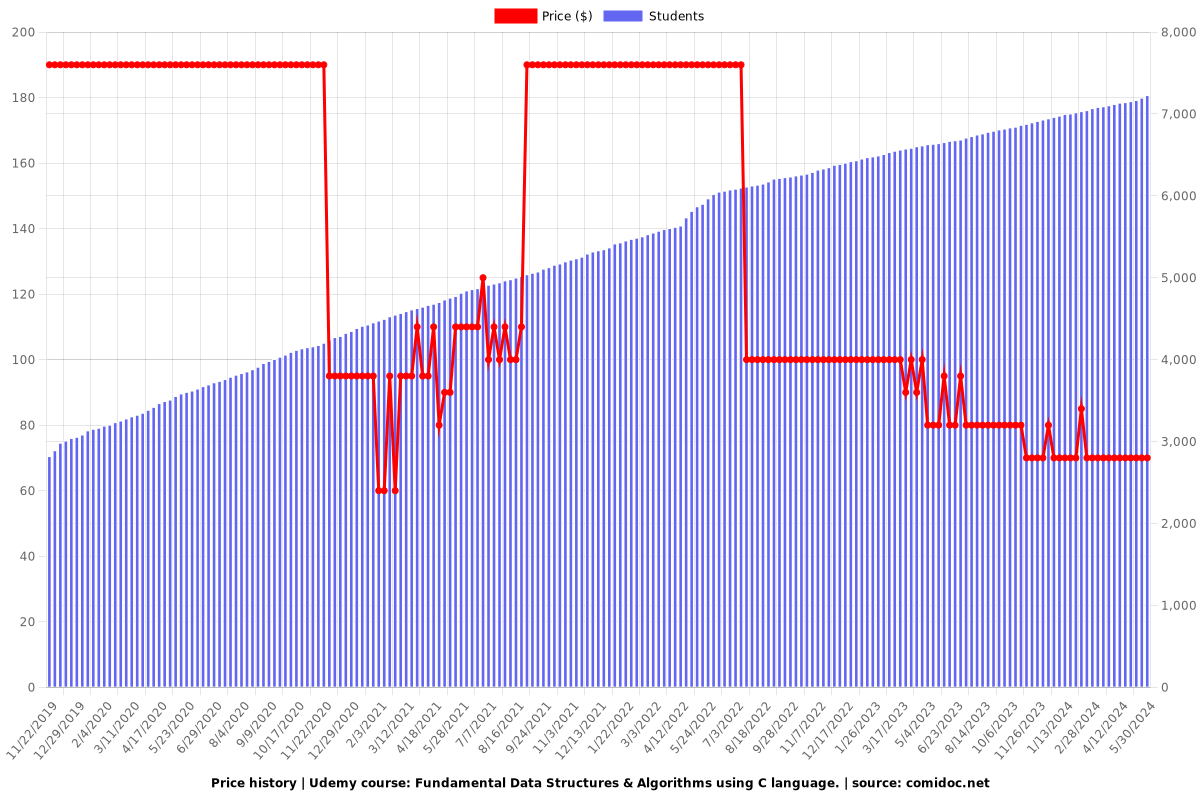
Rating
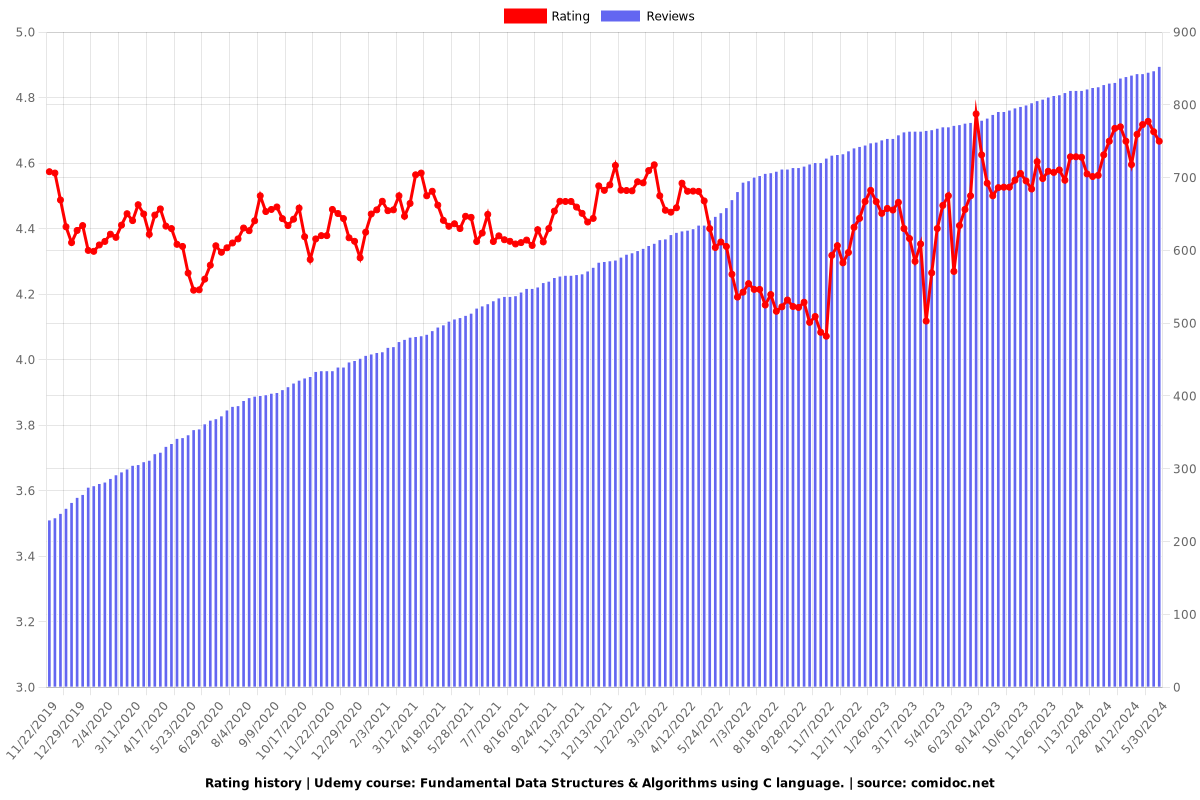
Enrollment distribution
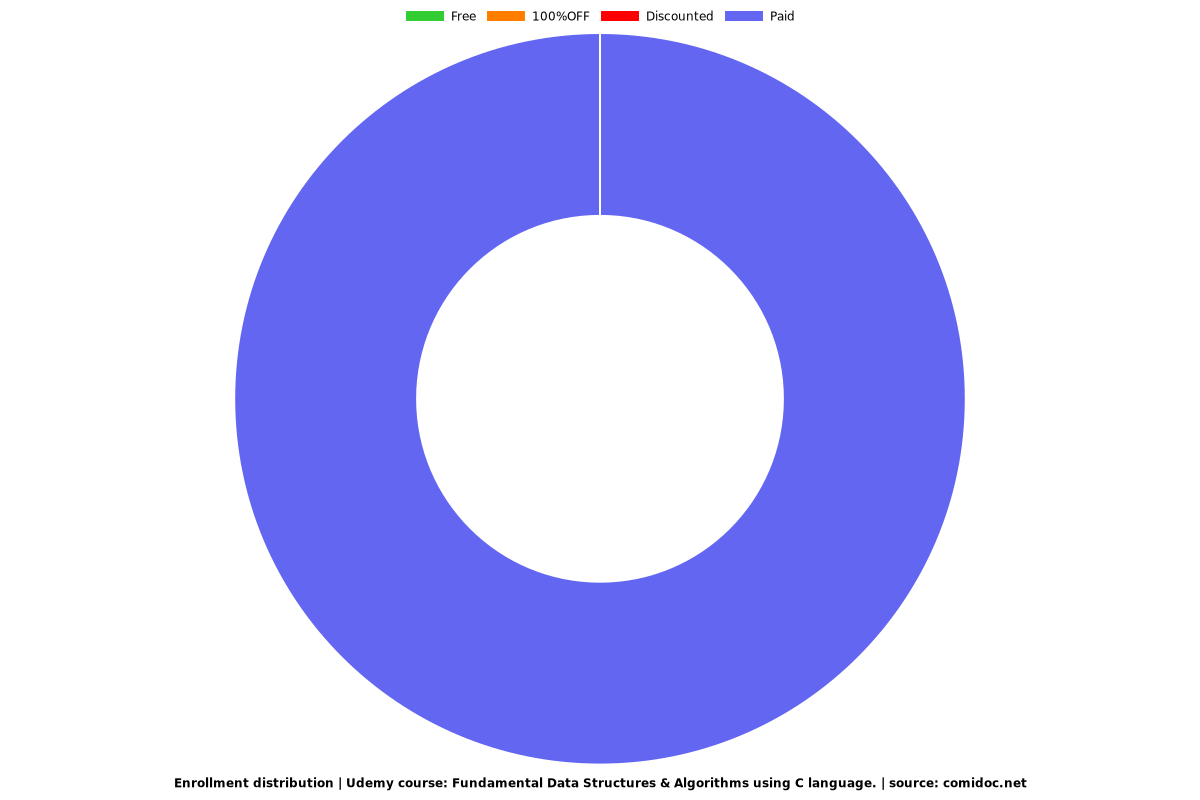