Data Structures and Algorithms | Coding Interview Q&A
Learn Data Structures & Algorithms from Scratch. + Master Coding Interviews . From basic to advanced.
3.72 (167 reviews)
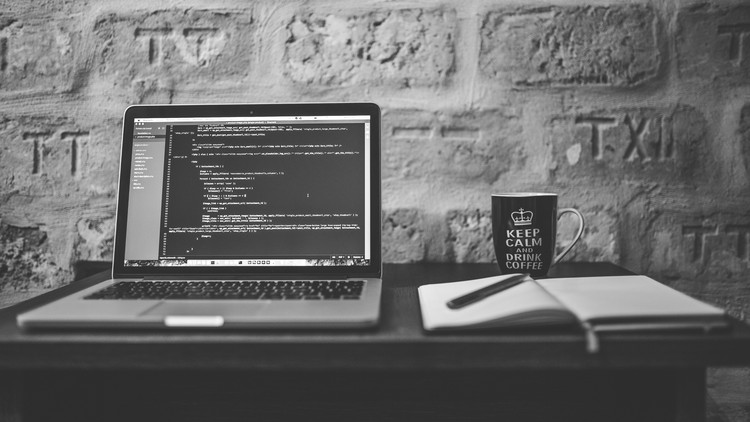
9,743
students
12.5 hours
content
Feb 2019
last update
$19.99
regular price
What you will learn
Master any coding interview in the largest tech companies in the world
Have an excellent understanding of how each Data Structure works and how to get the best out of them.
Know how to create by yourself the most efficient algorithms that can exist for any problem
Feel completely confident when talking about complex DS & Algorithms and how they work
Know how to implement each Data Structure from scratch.
Downloadable code of ALL the examples covered in this course
Screenshots
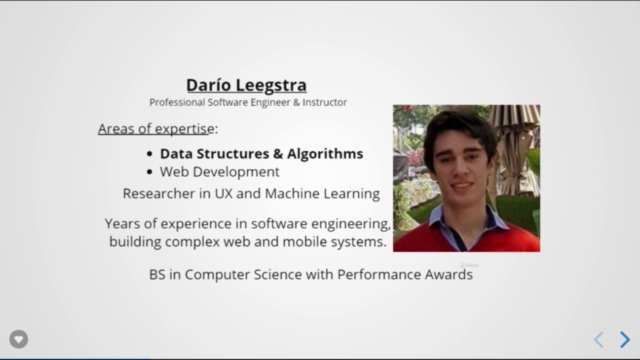
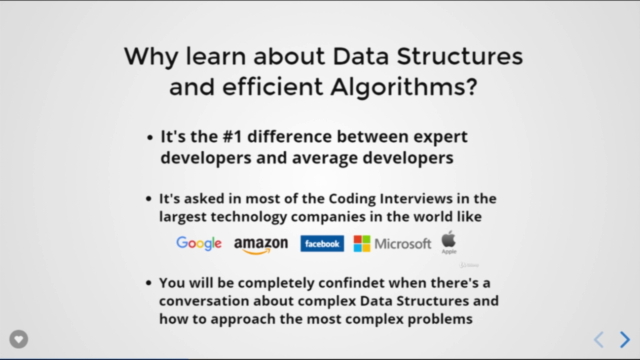
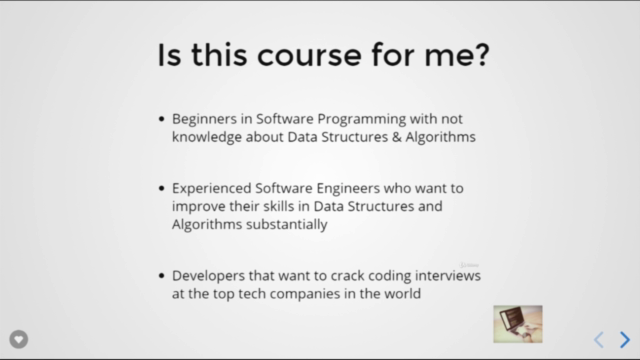
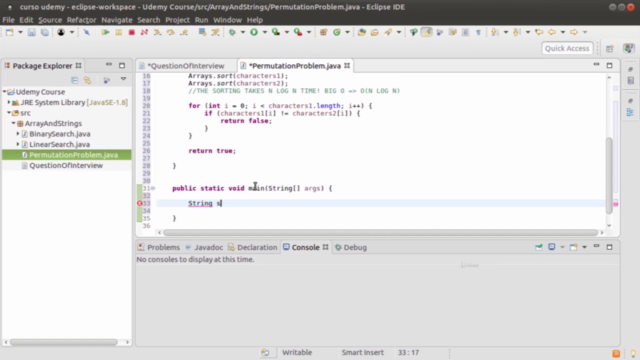
Related Topics
2052993
udemy ID
11/27/2018
course created date
6/17/2019
course indexed date
Bot
course submited by