C#/.NET - 50 Essential Interview Questions (Junior Level)
Get ready for your next interview! An in-depth guide to C# core concepts, SOLID principles and popular design patterns.
4.84 (725 reviews)
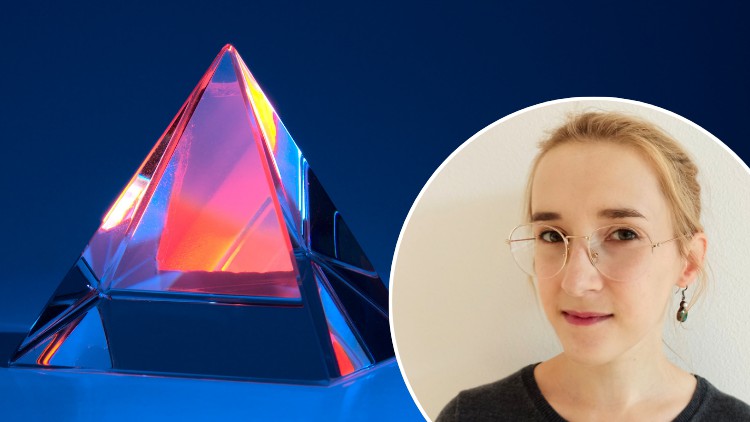
4,627
students
7 hours
content
Mar 2025
last update
$89.99
regular price
What you will learn
Prepare for 50 essential C# interview questions. With bonus questions, learn answers to 160 interview questions in total!
Master fundamental concepts of object-oriented programming.
Learn SOLID principles.
Get to know 5 popular design patterns.
Practice by solving in-browser coding exercises.
Get ready for tricky questions by gaining a deep understanding of C#-related topics.
Get a free e-book with all answers.
Screenshots
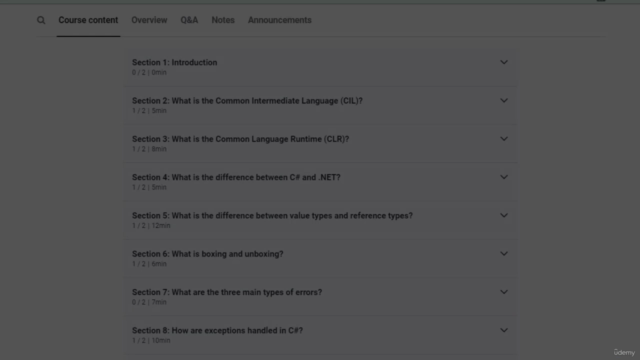
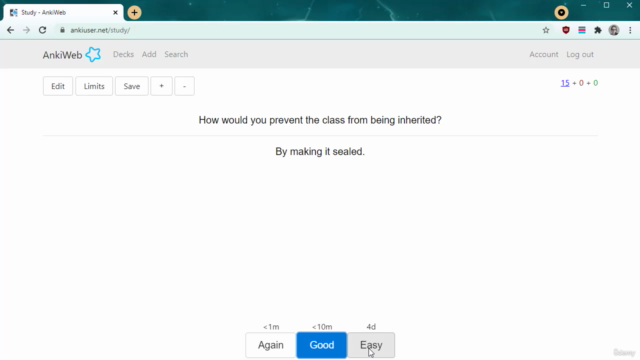
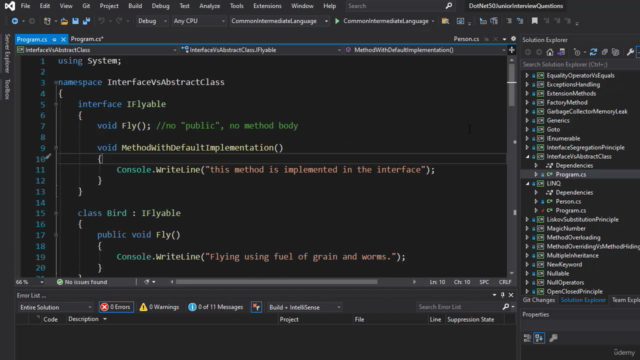
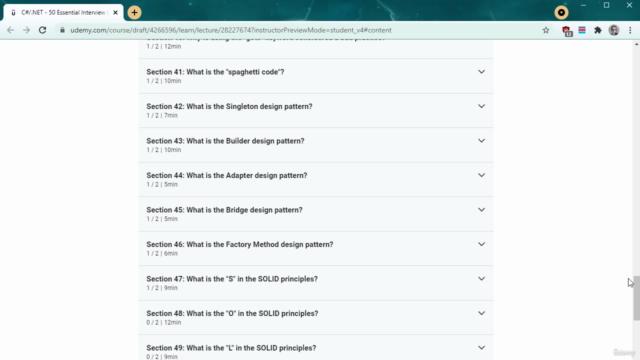
Related Topics
4266596
udemy ID
8/27/2021
course created date
9/6/2021
course indexed date
Bot
course submited by