Create ASP.Net Core Web API (step by step for beginners)
Build database driven CRUD API with C#, ASP .NET Core MVC and Entity Framework Core. Project based learning
4.57 (436 reviews)
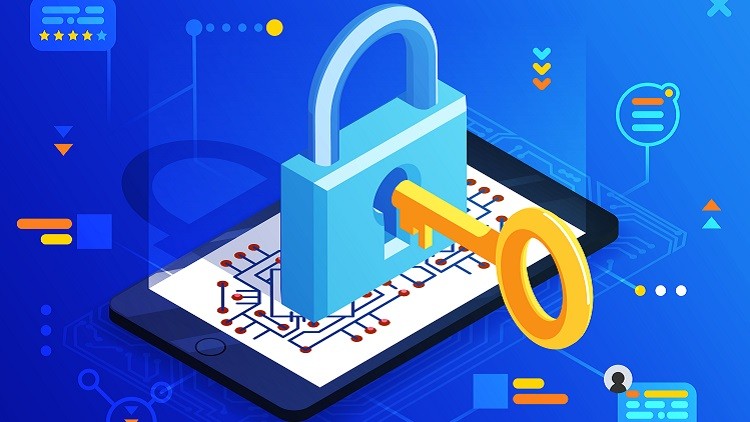
3,540
students
10 hours
content
Nov 2020
last update
$69.99
regular price
What you will learn
Creation of CRUD API in Asp .Net Core
.Net Core Services
Data Transfer Objects (DTO)
Use of DbContext for 1-to-many and many-to-many relationship
Understanding of Dependency Injection
Understanding of the use of Interfaces in C# and .Net Core
Screenshots
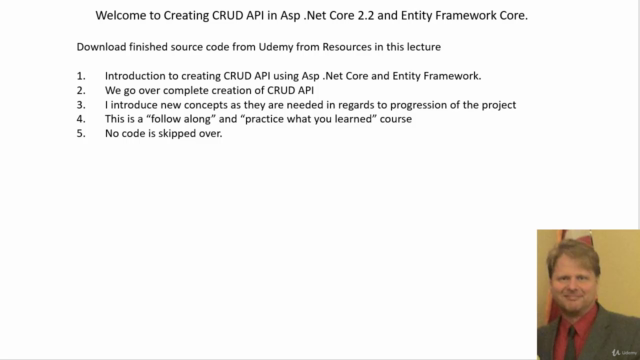
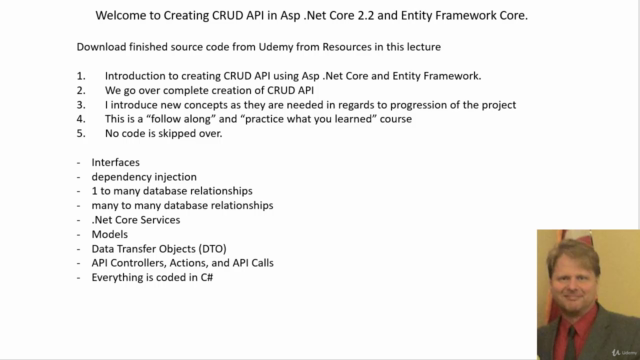
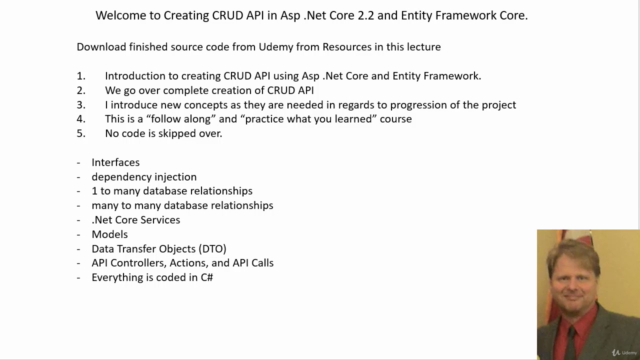
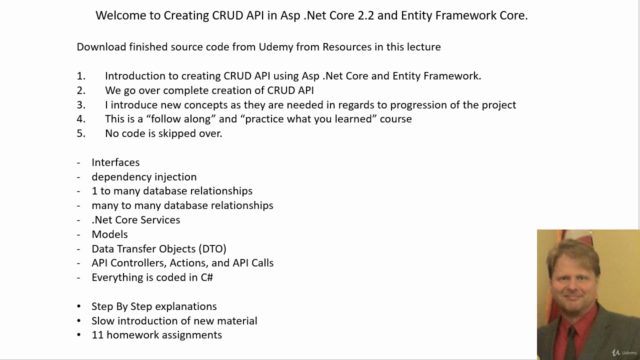
Related Topics
2306100
udemy ID
4/4/2019
course created date
5/13/2019
course indexed date
Bot
course submited by