Automated Software Testing with Python
Learn about automated software testing with Python, BDD, Selenium WebDriver, and Postman, focusing on web applications
4.43 (5624 reviews)
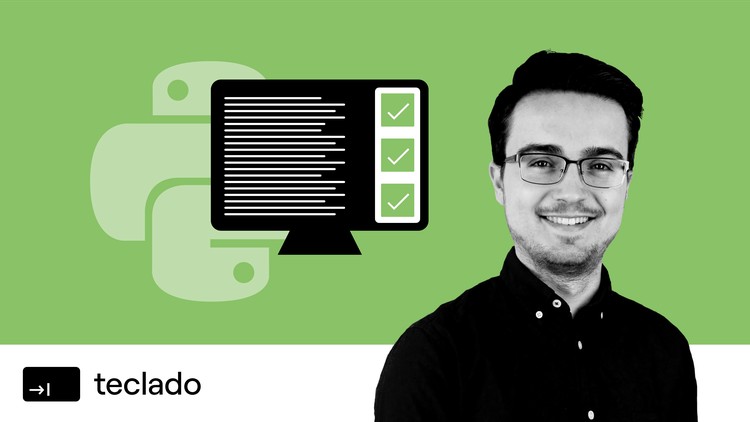
39,888
students
13.5 hours
content
Nov 2024
last update
$99.99
regular price
What you will learn
Everything you need to know about automated software testing with Python (and how to enjoy testing, too!)
Avoid common pitfalls and implement best practices when writing automated tests
Write complete system tests using Python and tools like Postman
Automate your application testing by setting up a continuous integration pipeline using Travis CI
Browser-based acceptance testing using Behave and Selenium WebDriver
Screenshots
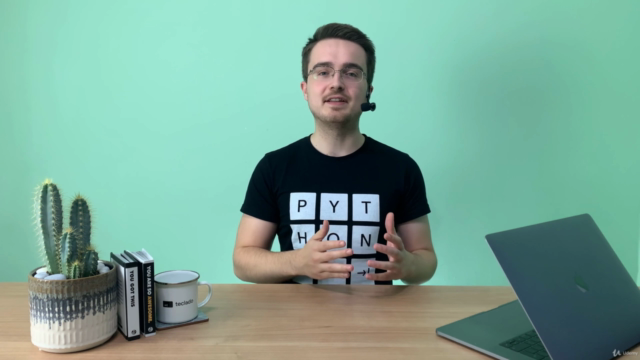
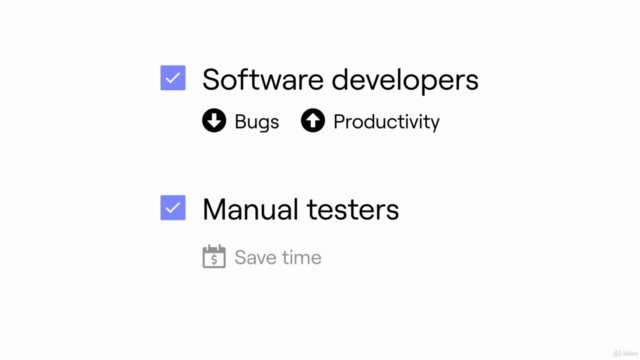
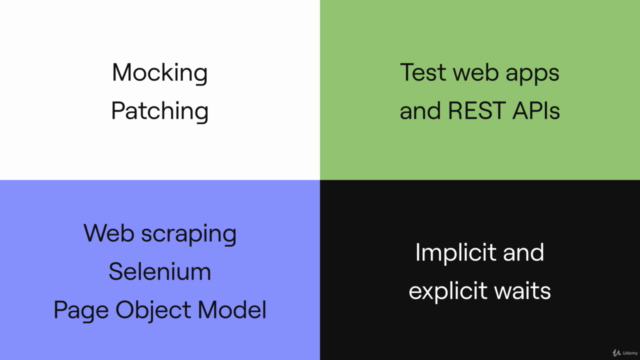
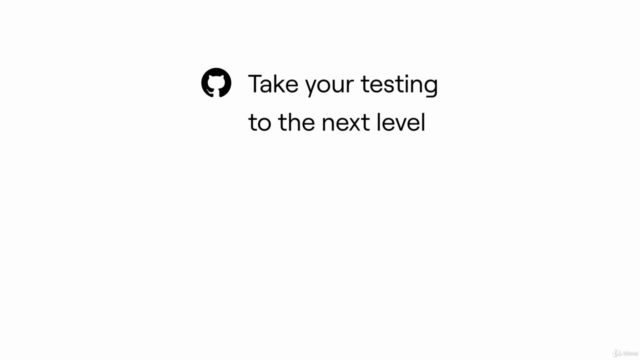
Related Topics
1319746
udemy ID
8/12/2017
course created date
9/12/2019
course indexed date
Bot
course submited by