ASP.NET Core - SOLID and Clean Architecture
Create a SOLID and testable ASP.NET Core Application using CQRS, Mediator Pattern and clean architecture.
4.62 (2217 reviews)
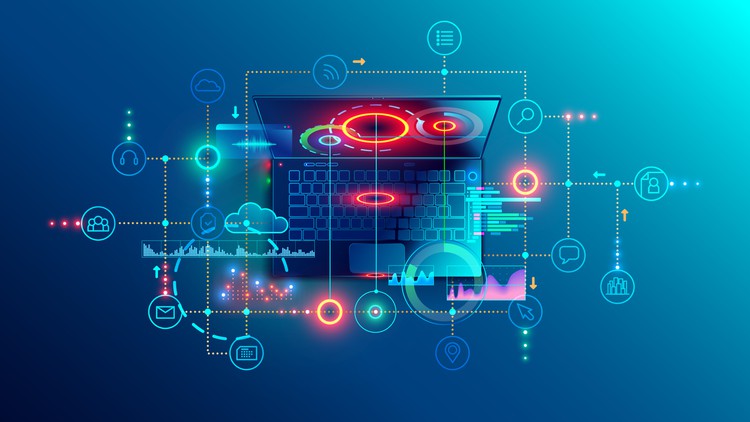
16,431
students
10 hours
content
Jan 2025
last update
$79.99
regular price
What you will learn
Implement SOLID Principles
ASP .NET Core Blazor and API Development
Advanced Tools - MediatR, Automapper, Fluent API and Validation
Custom Exceptions and Global Error Handling
Custom .NET Core Middleware
Using NSwag and NSwag Studio
Use Swagger for API Documentation
Implement CQRS Pattern
Use Identity and JWT To Secure Application API
Build API Client Secure Application
Moq and Shouldly Frameworks
Unit Testing
Related Topics
4003980
udemy ID
4/24/2021
course created date
8/18/2021
course indexed date
Bot
course submited by