Angular Testing Masterclass (Angular 19)
A complete guide to Angular 19 Unit Testing and End to End (E2E) Testing, including Testing best practices and CI
4.51 (6064 reviews)
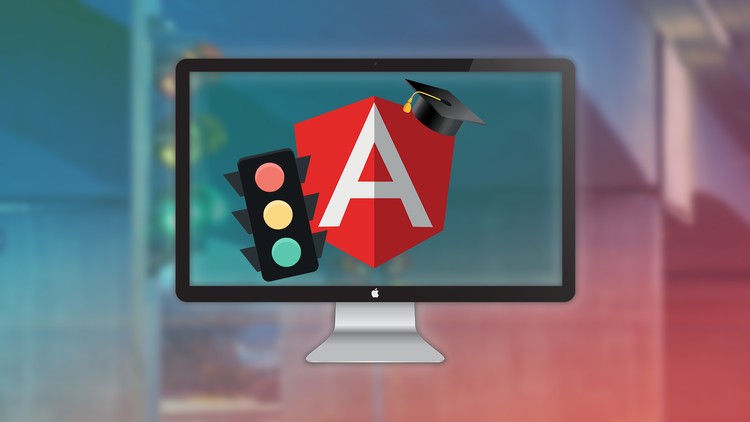
29,455
students
5 hours
content
Apr 2025
last update
$79.99
regular price
What you will learn
Code in Github repository with downloadable ZIP files per section
Testing Fundamentals
Angular Unit Testing Best Practices
Angular E2E Testing with Cypress
Angular Component and Service Testing
Asynchronous Angular Testing with fakeAsync and Async
Continuous Integration with Travis CI
Screenshots
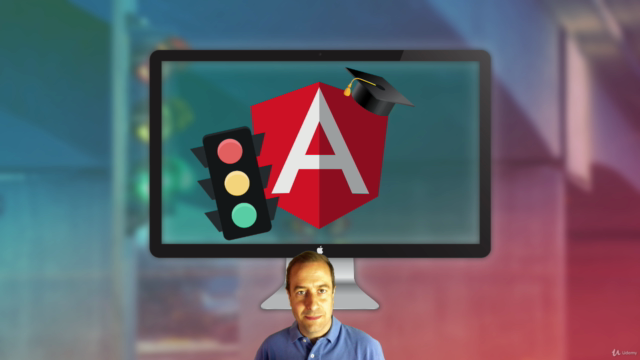
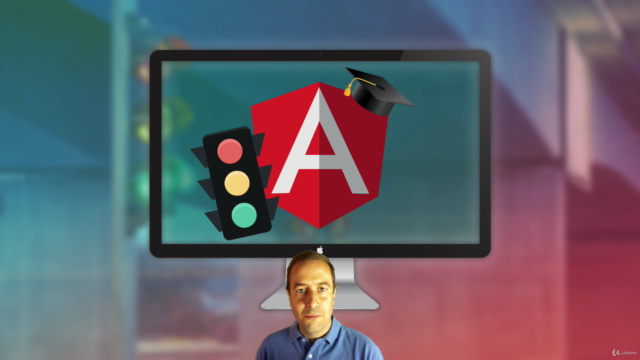
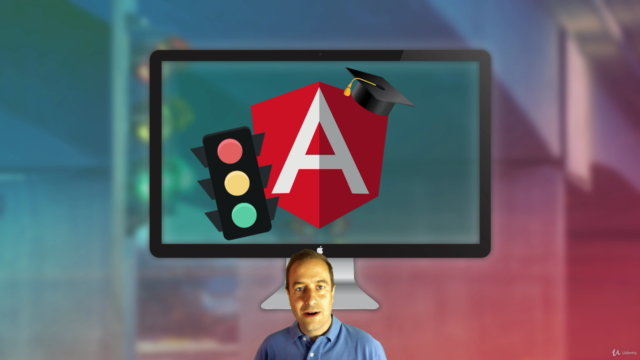
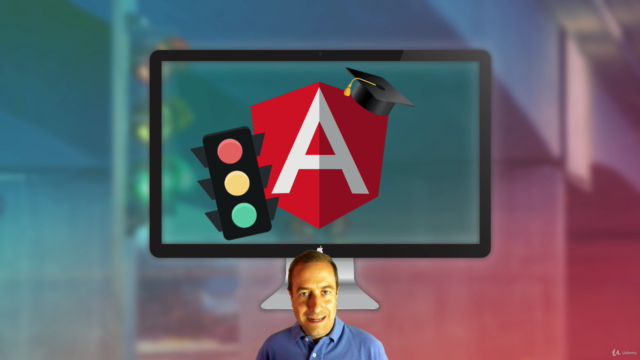
Related Topics
2308032
udemy ID
4/5/2019
course created date
11/20/2019
course indexed date
Bot
course submited by