Android app development for beginners (with Kotlin)
Kotlin Android Apps Development (Beginner to advanced)
4.42 (83 reviews)
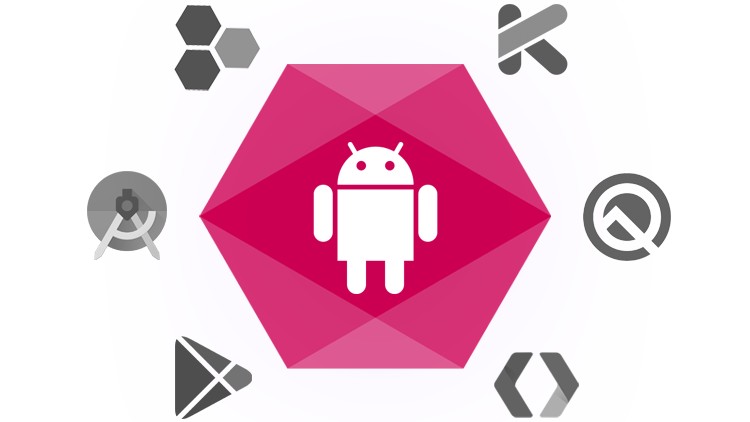
6,232
students
13 hours
content
Jun 2020
last update
$19.99
regular price
What you will learn
You will learn how to install tools
You will learn Kotlin for Android
You will learn how to develop Android apps
You will learn how to Implement 4 real apps
You will learn how to use database in your apps
You will learn how to publish your apps on Google Play
1- Installing and Setting Up Tools
Introcucing tools (IDE + Android SDK + Emulator + JDK)
How to download and install required tools
Creating a new Android Studio project
How to change the font of code editor
What is an activity
What is androidmanifest file
Developing your first Android app
Attributes panel
Code completion list
Displaying a toast
Running your app on an emulator
Examining different parts of an emulator
Send sms and phone calls between two emulators
Enabling directional pad
Cloud-based testing
Installing Google USB driver
Enabling developr options
Connecting a real device to the Android Studio
2- Kotlin _ Say Hello to Basics
Int data type
Declaring and initializing variables
Single line comment
var vs val
print() function
Floating point data type
Boolean data type
String data type
Type inference system
Naming conventions (Camel Case)
Data member and function member
dec() function
compareTo() function
Intellisence
Argument
replace(string) function
replace(char) function
char data type
toInt() function
toIntOrNull() function
NumberFormatException
toFloatOrNull() function
What is Null?
Kotlin Nullable types
Arithmetic operators
Operator precedence
Assignment operators
readLine() function
3- App ( Tip Calculator ) + Android Basic Views
TextView
Seekbar
EditText
Button
Set constraints for views
Resolving the "Hardcoded strings" warning
Converting text to number
Working with seekbar's listeners (setOnSeekBarChangeListener)
Concatenating strings, variables and expressions with the + operator
Concatenating strings, variables and expressions with String Templates
4- Kotlin _ Control Flow
If expression
If Else expression
Relational operators
Gutter area
Debugging the apps by using breakpoints
Transfer of control
How to check if a numbers is even or odd
Logcat tab
Toast messages
Statement vs Expression
lift out assignment
Any data type
When expression with arg
When vs If
When expression without arg
Combining concitions in a when expression
An app that determines if a number is prime number
How to hold a range of values (double dotted and in operators)
Using the when expression with ranges
Logical AND operator
Useful Android Studio shortcut keys
Defining range variables
5- Kotlin _ Functions
Simple functions
Functions with Parameters
Functions with return type
Function signature
Parameter vs Argument
Userful Android Studio shortcut keys
Multi line comment
6- Kotlin _ Immutable and Mutable Collections + Loops
Defining and initializing the Arrays
Using the arrays
Manipulating the array elements
Any data type
ArrayIndexOutOfBound exception
Data types and performance
How to define an array type explicitly
The second way of declaring and initializing an array
Iterating over arrays by using the for loop
Destructuring declaration
Iterate through a Range
How to get the numbers of an array elements
withIndex() function
Until keyword
listOf() function
mutableListOf() funciton
Array vs Collection
mutable vs immutable
7- Kotlin _ Classes + Enums + Exception Handling
Declaring classes
How to create objects
Constructors
Iterating through an array of objects
Vertical selection
Property and method
Class header
Primary constructor
Declaring properties from the primary constructor
Class diagram
How Enums make things easier
Happy path
Try Catch block
Multiple catch blocks
Finally block
8- App ( English Stories ) + Activities + List View + Singleton Pattern
Displaing data into a Listview
ListView click listener
How to creating a new Activity
Passing data between activities
Scrolling toolbar
How to set the start up Activity
FAB (Floating Action Button)
Snakbar
Singleton pattern
Private visibility modifier
Public visibility modifier
Overriding the functions
String controls characters
9- App ( My Notes ) + SQLite Database + Activity Life Cycle + Action Bar + Alert Dialog
Set the Margin attribute for views
Designing SQLite databases for Android apps (Fields, Records, Tables, ...)
Extending classes
SQLiteOpenHelperClass
TODO() functions
NullPointerException
Check null values
Safe call operator
Not null assertion operator
Using readble connetions to read data from database
Using writable connections to write data to database
OnDestroy() funciton
Performance tune-up while working ith databases
Variables' scope
Up button
<Meta Data> tag
How to add menu to Action Bar
Working with Asset studio
Refactoring resource names
XML namespaces
match-constraint attribute
Hint attribute
Setting EditText text attribute
Changing the EditText focus programatically
How to check if an EditText is empty
Inserting new records into the database
Up button vs Back button
Activity life cycle
Navigating clipboard items
Refactoring functions
How to close an Activity programatically
Alert Dialog
Updating and Deleting records from database
10- App ( Best Quotes ) + Recycler View +Card View + Intents + Guidelines + Unicode Characters
How to insert default values into the database
Designing layouts by using a Card View
Linear Layout
Image View
Layout Margin attribute
Writing a customized Adapter for Recycler View
Recycler View vs List View
Inner classes
findViewByID() function
Arranging and managing Recycler View items by using LayoutManager
Using the customized Adapter View
Passing functions in Kotlin as parameters
Guidelines in constraint layout
On item click listener for RecyclerView
Creating DB in memory
Explicit intent and Implicit intent
Ripple effect
Share data with other apps
11- App ( Animating a Spaceship + Best Quotes ) + Animation in Android + Splash Screen
Alpha animation
Translate animation
Rotate animation
Scale animation
Companion objects
Creating Splash Screens by using Lottie library
Using third-party libraries
Assets folder
Application context vs the this keyword
noHistory attribute
12- Publishing Your App
Parallex effect
Safe zone
Legacy icons
Adaptive icons
Debug key vs Release key
Signing the APK
Screenshots
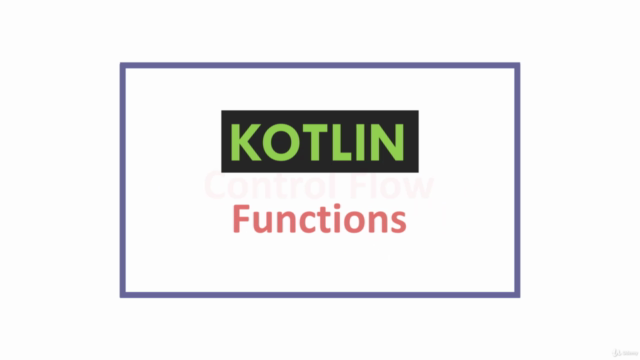
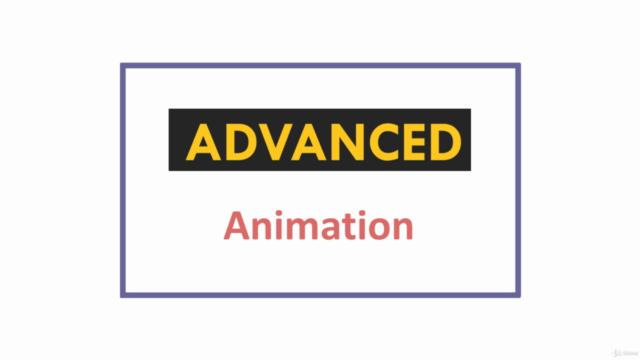
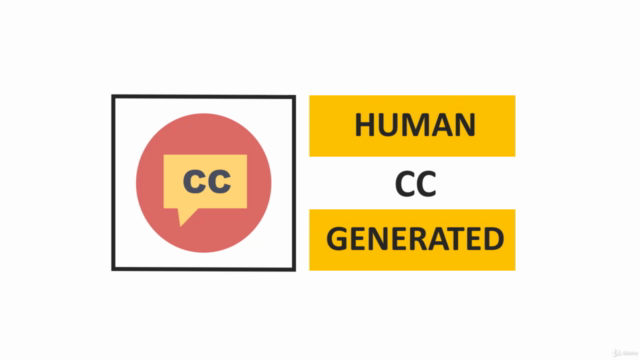
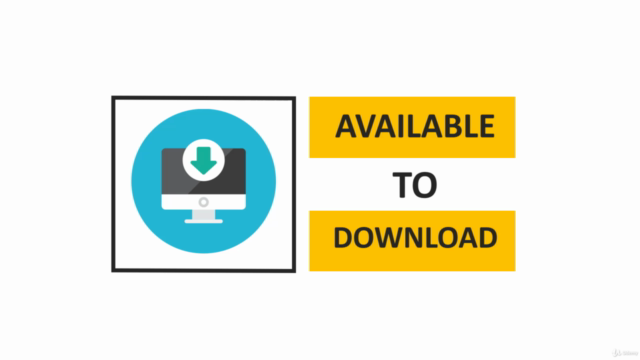
Related Topics
2328398
udemy ID
4/18/2019
course created date
11/21/2019
course indexed date
Bot
course submited by