Python NumPy Programming and Project Development
Expert-level Python programming with NumPy tutorials. Apply NumPy concepts to develop real-time projects & applications.
4.07 (27 reviews)
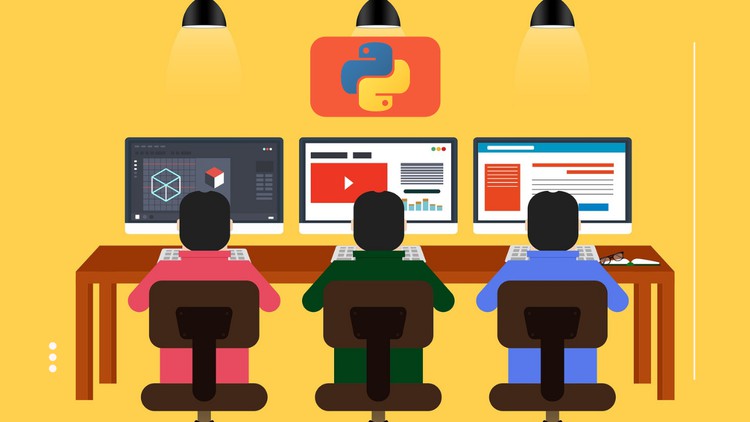
6,069
students
24.5 hours
content
May 2024
last update
$19.99
regular price
What you will learn
Advanced Python programming with NumPy concepts and its application
NumPy Module Projects - 6 full tutorials on project implementation using NumPy
NumPy - Ndarray Object
NumPy - Array Attributes
NumPy - Array Creation Routines
NumPy - Array from Numerical Ranges
NumPy - Advanced Indexing
NumPy – Broadcasting
NumPy - Iterating over Array
NumPy - Array Manipulation
NumPy - Binary Operators
NumPy - String Functions
NumPy - Mathematical Functions
NumPy - Arithmetic Operations
NumPy - Statistical Functions
NumPy - Sort, Search & Counting Functions
NumPy - Copies & Views
NumPy - Matrix Library
NumPy - Linear Algebra
Related Topics
3498694
udemy ID
9/14/2020
course created date
10/5/2020
course indexed date
AhmedELKING
course submited by