Advanced Algorithms and Data Structures in Python
Fenwick trees, Caches, Splay Trees, Prefix Trees (Tries), Substring-Search Algorithms and Travelling Salesman Problem
4.67 (65 reviews)
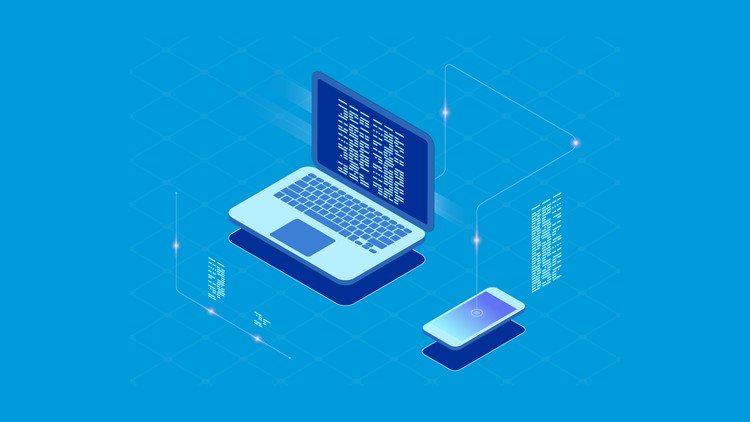
3,170
students
10.5 hours
content
Jan 2022
last update
$13.99
regular price
What you will learn
Have a good grasp of algorithmic thinking
Be able to develop your own algorithms
Be able to detect and correct inefficient code snippets
Understand Fenwick trees
Understand caches (LRU caches and Splay Trees)
Understand tries and ternary search trees
Understand substring search algorithms (Rabin-Karp method, KMP algorithm and Z algorithm)
Understand the Hamiltonian cycle problem (and travelling salesman problem)
Understand Eulerian cycle problem
Related Topics
1985884
udemy ID
10/24/2018
course created date
11/16/2019
course indexed date
Bot
course submited by