50 popular coding interview problems
Prepare for your coding interview with these 50 solved and explained popular coding problems
4.40 (358 reviews)
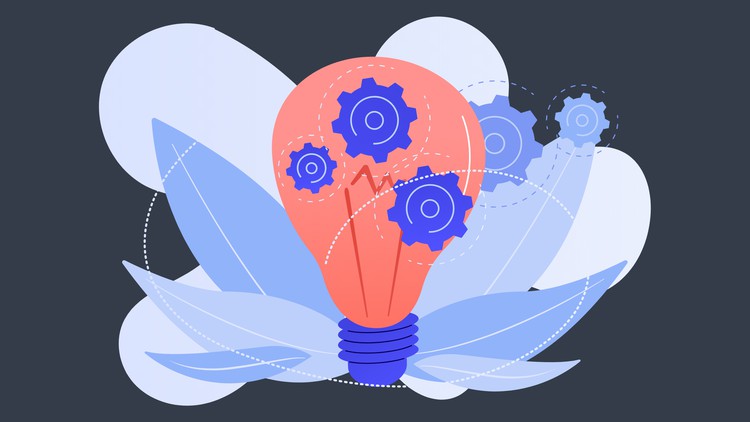
6,971
students
8.5 hours
content
Apr 2022
last update
$79.99
regular price
What you will learn
How to solve coding interview problems
How to use recursion, dynamic programming, memoization, divide and conquer, backtracking...
How to solve problems related to various data structures
Screenshots
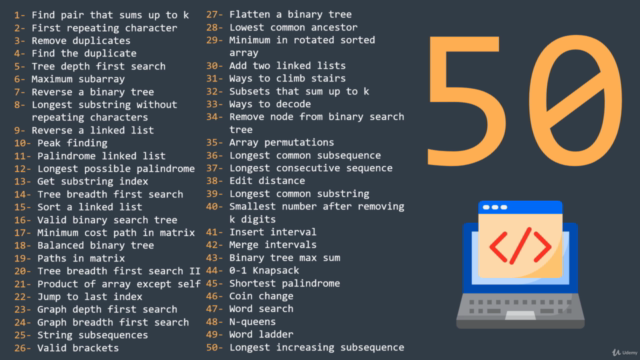
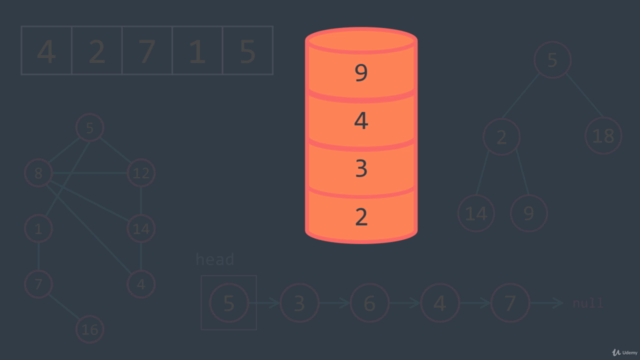
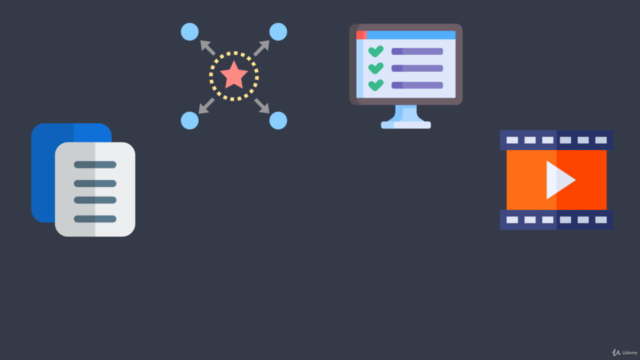
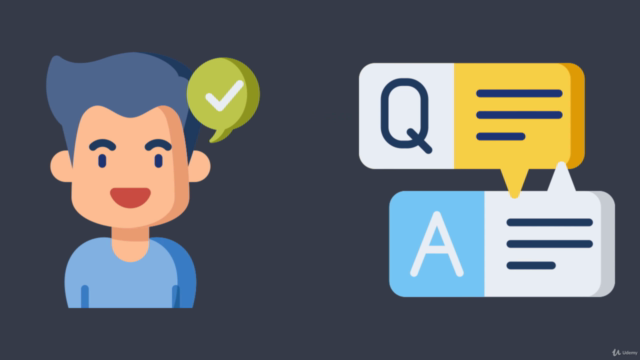
Related Topics
3411244
udemy ID
8/11/2020
course created date
8/31/2020
course indexed date
Bot
course submited by